Firebase Storage
Add to favorites
Upload, delete and list files in Firebase Storage
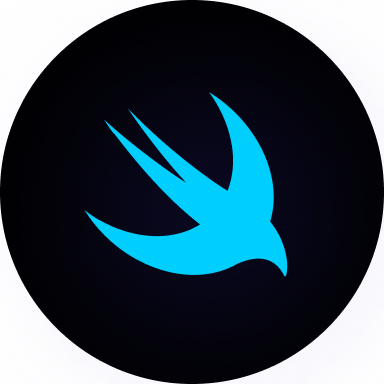
SwiftUI Advanced Handbook
1
Firebase Auth
8:18
2
Read from Firestore
8:01
3
Write to Firestore
5:35
4
Join an Array of Strings
3:33
5
Data from JSON
5:08
6
HTTP Request
6:31
7
WKWebView
5:25
8
Code Highlighting in a WebView
5:11
9
Test for Production in the Simulator
1:43
10
Debug Performance in a WebView
1:57
11
Debug a Crash Log
2:22
12
Simulate a Bad Network
2:11
13
Archive a Build in Xcode
1:28
14
Apollo GraphQL Part I
6:21
15
Apollo GraphQL Part 2
6:43
16
Apollo GraphQL Part 3
5:08
17
Configuration Files in Xcode
4:35
18
App Review
5:43
19
ImagePicker
5:06
20
Compress a UIImage
3:32
21
Firebase Storage
11:11
22
Search Feature
9:13
23
Push Notifications Part 1
5:59
24
Push Notifications Part 2
6:30
25
Push Notifications Part 3
6:13
26
Network Connection
6:49
27
Download Files Locally Part 1
6:05
28
Download Files Locally Part 2
6:02
29
Offline Data with Realm
10:20
30
HTTP Request with Async Await
6:11
31
Xcode Cloud
9:23
32
SceneStorage and TabView
3:52
33
Network Connection Observer
4:37
34
Apollo GraphQL Caching
9:42
35
Create a model from an API response
5:37
36
Multiple type variables in Swift
4:23
37
Parsing Data with SwiftyJSON
9:36
38
ShazamKit
12:38
39
Firebase Remote Config
9:05
Add Firebase to your project
If you don't already have Firebase in your project, read the Firebase Auth section of this handbook to learn how to enable it. After you've added the Firebase Swift Package (https://github.com/firebase/firebase-ios-sdk.git) to your project, remember to check the FirebaseStorage library option.
Create a StorageManager
Create a StorageManager.swift file and import SwiftUI and Firebase at the top.
import SwiftUI
import Firebase
After the import, we'll create a class that will conform to an ObservableObject and initialize Firebase Storage.
class StorageManager: ObservableObject {
let storage = Storage.storage()
}
Create a function to upload
You can easily upload a UIImage to Firebase Storage with the putData:metadata:completion: method from Firebase.
Let's start by creating our function. It'll take an image as an argument, which is of UIImage type.
func upload(image: UIImage) {
}
Inside the function, we need to create a reference. A reference is the path in which we will save the photo. Here, we want to save the photo under the images folder, and rename the file image.jpg.
// Create a storage reference
let storageRef = storage.reference().child("images/image.jpg")
Next, we need to compress and convert the image passed to our function into a JPEG file. Read more about it in the Compress a UIImage section of this handbook.
// Resize the image to 200px in height with a custom extension
let resizedImage = image.aspectFittedToHeight(200)
// Convert the image into JPEG and compress the quality to reduce its size
let data = resizedImage.jpegData(compressionQuality: 0.2)
Change the type of file we're going to upload in Firebase Storage's metadata. If you don't change it, the file saved in Firebase Storage will be of type application/octet-stream.
let metadata = StorageMetadata()
metadata.contentType = "image/jpg"
Lastly, we'll upload the image using the putData:metadata:completion: method from Firebase.
if let data = data {
storageRef.putData(data, metadata: metadata) { (metadata, error) in
if let error = error {
print("Error while uploading file: ", error)
}
if let metadata = metadata {
print("Metadata: ", metadata)
}
}
}
Since the .jpegData(compressionQuality:) function returns an optional Data (Data?), we need to unwrap that optional with an if statement. If there's an error, we'll print it in the console. If all go as planned, the metadata should be printed in the console.
List items
You can list all the items in your Firebase Storage with the listAll(completion:) method from Firebase. You can also call the list(withMaxResults:completion:) to list a limited amount of files from your database.
Let's create our listAllFiles function.
func listAllFiles() {
}
Create a storage reference. We want to list all files from the images folder.
let storageRef = storage.reference().child("images")
Then, just call the listAll function from Firebase. If there's an error, we'll print it. If there's no error, we'll print each item we get from the images folder in Firebase Storage.
storageRef.listAll { (result, error) in
if let error = error {
print("Error while listing all files: ", error)
}
for item in result.items {
print("Item in images folder: ", item)
}
}
You can also list just one item with the list(withMaxResults:completion:) method:
func listItem() {
// Create a reference
let storageRef = storage.reference().child("images")
// Create a completion handler - aka what the function should do after it listed all the items
let handler: (StorageListResult, Error?) -> Void = { (result, error) in
if let error = error {
print("error", error)
}
let item = result.items
print("item: ", item) // Returns an array of items
}
// List the items
storageRef.list(maxResults: 1, completion: handler)
}
Delete an item
To delete an item, you can use the listItem() function we just create to get the storage reference of the item you want to delete.
func deleteItem(item: StorageReference) {
item.delete { error in
if let error = error {
print("Error deleting item", error)
}
}
}
Update rules
Don't forget to update your Firebase Storage rules to allow users to read and write to the Storage. Navigate to the Firebase Console > Storage > Rules and update the rules depending on your app's needs.
Use your functions in your Views
To use your functions in your View, simply create a new instance of StorageManager at the top of the file.
var storageManager = StorageManager()
Then, you can call all the functions we created in StorageManager.swift on the storageManager variable we just created in the View. For example, I can listen to the change of an image and upload it to Firebase Storage:
.onChange(of: image, perform: { image in
storageManager.upload(image: image)
})
Or you can list all items in Firebase Storage onAppear:
.onAppear {
storageManager.listAllFiles()
}
Final code
Here is the final code of our StorageManager file:
import SwiftUI
import Firebase
public class StorageManager: ObservableObject {
let storage = Storage.storage()
func upload(image: UIImage) {
// Create a storage reference
let storageRef = storage.reference().child("images/image.jpg")
// Resize the image to 200px with a custom extension
let resizedImage = image.aspectFittedToHeight(200)
// Convert the image into JPEG and compress the quality to reduce its size
let data = resizedImage.jpegData(compressionQuality: 0.2)
// Change the content type to jpg. If you don't, it'll be saved as application/octet-stream type
let metadata = StorageMetadata()
metadata.contentType = "image/jpg"
// Upload the image
if let data = data {
storageRef.putData(data, metadata: metadata) { (metadata, error) in
if let error = error {
print("Error while uploading file: ", error)
}
if let metadata = metadata {
print("Metadata: ", metadata)
}
}
}
}
func listAllFiles() {
// Create a reference
let storageRef = storage.reference().child("images")
// List all items in the images folder
storageRef.listAll { (result, error) in
if let error = error {
print("Error while listing all files: ", error)
}
for item in result.items {
print("Item in images folder: ", item)
}
}
}
func listItem() {
// Create a reference
let storageRef = storage.reference().child("images")
// Create a completion handler - aka what the function should do after it listed all the items
let handler: (StorageListResult, Error?) -> Void = { (result, error) in
if let error = error {
print("error", error)
}
let item = result.items
print("item: ", item)
}
// List the items
storageRef.list(maxResults: 1, completion: handler)
}
// You can use the listItem() function above to get the StorageReference of the item you want to delete
func deleteItem(item: StorageReference) {
item.delete { error in
if let error = error {
print("Error deleting item", error)
}
}
}
}
Learn with videos and source files. Available to Pro subscribers only.
Purchase includes access to 50+ courses, 320+ premium tutorials, 300+ hours of videos, source files and certificates.
Templates and source code
Download source files
Download the videos and assets to refer and learn offline without interuption.
Design template
Source code for all sections
Video files, ePub and subtitles
Videos
Assets
ePub
Subtitles
1
Firebase Auth
How to install Firebase authentification to your Xcode project
8:18
2
Read from Firestore
Install Cloud Firestore in your application to fetch and read data from a collection
8:01
3
Write to Firestore
Save the data users input in your application in a Firestore collection
5:35
4
Join an Array of Strings
Turn your array into a serialized String
3:33
5
Data from JSON
Load data from a JSON file into your SwiftUI application
5:08
6
HTTP Request
Create an HTTP Get Request to fetch data from an API
6:31
7
WKWebView
Integrate an HTML page into your SwiftUI application using WKWebView and by converting Markdown into HTML
5:25
8
Code Highlighting in a WebView
Use Highlight.js to convert your code blocks into beautiful highlighted code in a WebView
5:11
9
Test for Production in the Simulator
Build your app on Release scheme to test for production
1:43
10
Debug Performance in a WebView
Enable Safari's WebInspector to debug the performance of a WebView in your application
1:57
11
Debug a Crash Log
Learn how to debug a crash log from App Store Connect in Xcode
2:22
12
Simulate a Bad Network
Test your SwiftUI application by simulating a bad network connection with Network Link Conditionner
2:11
13
Archive a Build in Xcode
Archive a build for beta testing or to release in the App Store
1:28
14
Apollo GraphQL Part I
Install Apollo GraphQL in your project to fetch data from an API
6:21
15
Apollo GraphQL Part 2
Make a network call to fetch your data and process it into your own data type
6:43
16
Apollo GraphQL Part 3
Display the data fetched with Apollo GraphQL in your View
5:08
17
Configuration Files in Xcode
Create configuration files and add variables depending on the environment - development or production
4:35
18
App Review
Request an app review from your user for the AppStore
5:43
19
ImagePicker
Create an ImagePicker to choose a photo from the library or take a photo from the camera
5:06
20
Compress a UIImage
Compress a UIImage by converting it to JPEG, reducing its size and quality
3:32
21
Firebase Storage
Upload, delete and list files in Firebase Storage
11:11
22
Search Feature
Implement a search feature to filter through your content in your SwiftUI application
9:13
23
Push Notifications Part 1
Set up Firebase Cloud Messaging as a provider server to send push notifications to your users
5:59
24
Push Notifications Part 2
Create an AppDelegate to ask permission to send push notifications using Apple Push Notifications service and Firebase Cloud Messaging
6:30
25
Push Notifications Part 3
Tie everything together and test your push notifications feature in production
6:13
26
Network Connection
Verify the network connection of your user to perform tasks depending on their network's reachability
6:49
27
Download Files Locally Part 1
Download videos and files locally so users can watch them offline
6:05
28
Download Files Locally Part 2
Learn how to use the DownloadManager class in your views for offline video viewing
6:02
29
Offline Data with Realm
Save your SwiftUI data into a Realm so users can access them offline
10:20
30
HTTP Request with Async Await
Create an HTTP get request function using async await
6:11
31
Xcode Cloud
Automate workflows with Xcode Cloud
9:23
32
SceneStorage and TabView
Use @SceneStorage with TabView for better user experience on iPad
3:52
33
Network Connection Observer
Observe the network connection state using NWPathMonitor
4:37
34
Apollo GraphQL Caching
Cache data for offline availability with Apollo GraphQL
9:42
35
Create a model from an API response
Learn how to create a SwiftUI model out of the response body of an API
5:37
36
Multiple type variables in Swift
Make your models conform to the same protocol to create multiple type variables
4:23
37
Parsing Data with SwiftyJSON
Make API calls and easily parse data with this JSON package
9:36
38
ShazamKit
Build a simple Shazam clone and perform music recognition
12:38
39
Firebase Remote Config
Deliver changes to your app on the fly remotely
9:05
Meet the instructor
We all try to be consistent with our way of teaching step-by-step, providing source files and prioritizing design in our courses.
Stephanie Diep
iOS and Web developer
Developing web and mobile applications while learning new techniques everyday
7 courses - 36 hours
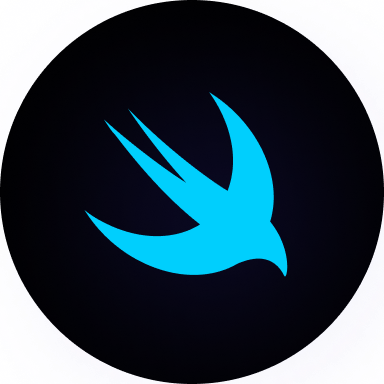
Build Quick Apps with SwiftUI
Apply your Swift and SwiftUI knowledge by building real, quick and various applications from scratch
11 hrs
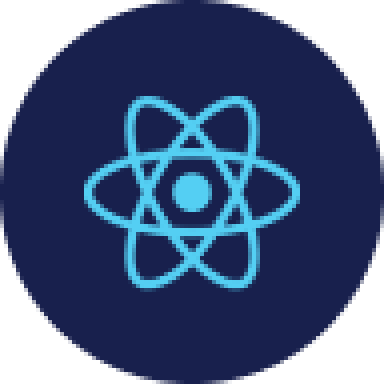
Advanced React Hooks Handbook
An extensive series of tutorials covering advanced topics related to React hooks, with a main focus on backend and logic to take your React skills to the next level
3 hrs
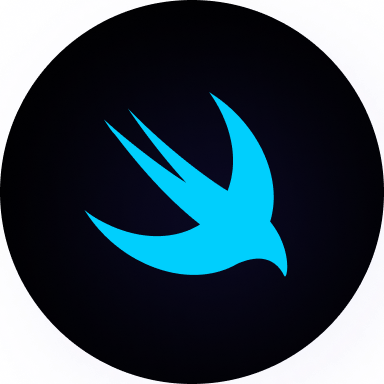
SwiftUI Concurrency
Concurrency, swipe actions, search feature, AttributedStrings and accessibility were concepts discussed at WWDC21. This course explores all these topics, in addition to data hosting in Contentful and data fetching using Apollo GraphQL
3 hrs
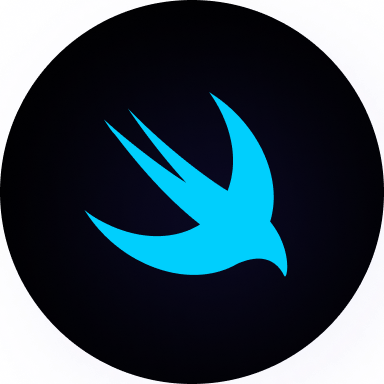
SwiftUI Combine and Data
Learn about Combine, the MVVM architecture, data, notifications and performance hands-on by creating a beautiful SwiftUI application
3 hrs
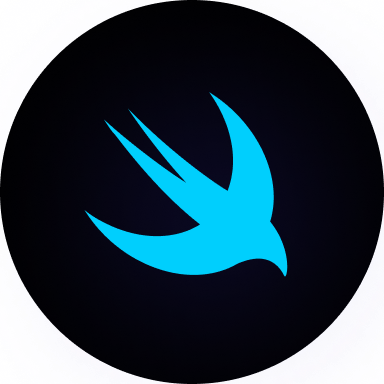
SwiftUI Advanced Handbook
An extensive series of tutorials covering advanced topics related to SwiftUI, with a main focus on backend and logic to take your SwiftUI skills to the next level
4 hrs
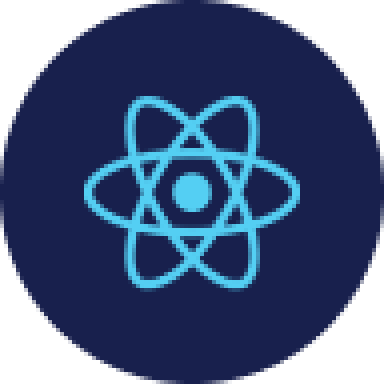
React Hooks Handbook
An exhaustive catalog of React tutorials covering hooks, styling and some more advanced topics
5 hrs
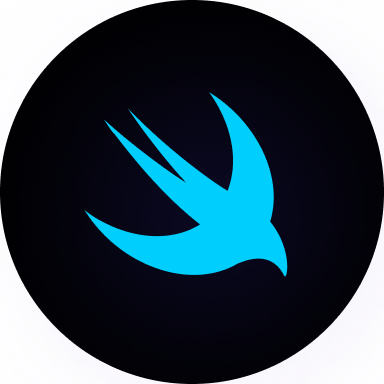
SwiftUI Handbook
A comprehensive series of tutorials covering Xcode, SwiftUI and all the layout and development techniques
7 hrs