Swift Sets
Add to favorites
Mastering Unique Collections with Fast Lookups and Powerful Operations
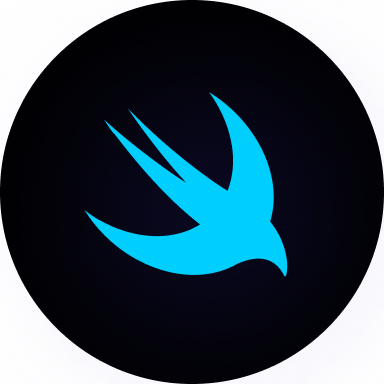
SwiftUI Fundamentals Handbook
1
Building Your iOS Development Foundation
2
SwiftUI Print Debugging
18:04
3
Comments Documentation Waypoints
22:02
4
Variables and Constants
11:37
5
Strings and Interpolation
13:22
6
Swift Operators: The Foundation of SwiftUI Logic
5:34
7
Swift Unary Operators
15:00
8
Swift Binary Operators
3:36
9
Arithmetic Operators
6:11
10
If-Else and Comparison Operators
12:32
11
Logical Operators
6:03
12
Ternary Operators
6:22
13
Blocks and Scope
10:22
14
Swift Collections: Arrays, Sets, and Dictionaries Overview
7:00
15
Swift Arrays
8:54
16
Swift Sets
11:03
17
Swift Dictionaries
18
For-Loops and Range
19
Optionals
20
Functions
1. Introduction to Sets
Sets are one of Swift's essential collection types, designed specifically for storing unique values in an unordered collection.
A. What is a Set?
i. Definition: A Set is an unordered collection of unique values of the same type. ii. Purpose: Sets help you store values without duplicates and test for membership efficiently. iii. Key characteristics: Unlike arrays, Sets have no defined order and cannot contain duplicate elements. iv. Memory representation: Sets use hash tables behind the scenes, making lookups extremely fast (O(1) complexity).
B. Why Use Sets?
i. Uniqueness enforcement: Automatically prevent duplicates in your collection. ii. Fast membership testing: Quickly check if an element exists in the collection. iii. Set operations: Perform mathematical set operations like union, intersection, and difference. iv. Performance optimization: Use Sets when order doesn't matter but uniqueness and lookup speed do. v. Data deduplication: Easily remove duplicates from collections.
2. Creating Sets in Swift
A. Set Literals
i. Using array literals with the Set type: The most common way to create a set.
let colors: Set<String> = ["Red", "Green", "Blue"]
// Duplicate elements are automatically removed
let numbersWithDuplicates: Set<Int> = [1, 2, 2, 3, 3, 3]
// Result: {1, 2, 3} (order not guaranteed)
ii. Empty sets: Creating sets with no elements.
// Method 1: Type annotation with empty array literal
let emptySet: Set<Int> = []
// Method 2: Using initializer syntax
let anotherEmptySet = Set<String>()
B. Set Type Annotation
i. Explicit type declaration: Declaring the type of elements a set will contain.
let vowels: Set<Character> = ["a", "e", "i", "o", "u"]
// For more complex types
let userSet: Set<(name: String, age: Int)> = [
(name: "Alice", age: 28),
(name: "Bob", age: 34)
]
ii. Set conversion: Creating sets from other collection types.
let numbersArray = [1, 2, 3, 4, 2, 3]
let numbersSet = Set(numbersArray) // {1, 2, 3, 4}
// From a string (creates a set of characters)
let letters = Set("Mississippi") // {"M", "i", "p", "s"}
iii. Sets of custom types: Creating sets of your own defined types.
struct User: Hashable {
let id: Int
let name: String
// Swift can automatically synthesize the Hashable conformance
// for simple structs, but you can customize it if needed
}
let users: Set<User> = [
User(id: 1, name: "Alice"),
User(id: 2, name: "Bob")
]
3. Accessing Set Elements
A. Basic Access Methods
i. Checking if a set contains a specific element: Fast membership testing.
let fruits: Set<String> = ["Apple", "Banana", "Orange"]
let hasApple = fruits.contains("Apple") // true
let hasMango = fruits.contains("Mango") // false
ii. Retrieving elements: Unlike arrays, sets don't support direct index access.
// You can access elements through iteration
for fruit in fruits {
print(fruit) // Order not guaranteed
}
// Or convert to an array if needed
let fruitsArray = Array(fruits)
B. Properties and Methods for Access
i. first
property: Get an arbitrary element from the set.
if let anyFruit = fruits.first {
print("A fruit from the set: \(anyFruit)")
}
// Note: Since sets are unordered, "first" just means "any element"
**ii. randomElement()
: Get a random element from the set.
if let randomFruit = fruits.randomElement() {
print("Random selection: \(randomFruit)")
}
iii. Accessing elements matching conditions: Finding elements that satisfy specific criteria.
let longFruit = fruits.first { $0.count > 5 }
4. Modifying Sets
A. Adding Elements
**i. insert(_:)
: Add an element to the set (if it doesn't already exist).
var vegetables: Set<String> = ["Carrot", "Broccoli"]
let insertResult = vegetables.insert("Spinach")
// vegetables is now {"Carrot", "Broccoli", "Spinach"}
print(insertResult.inserted) // true
print(insertResult.memberAfterInsert) // "Spinach"
// Trying to insert an existing element
let duplicateResult = vegetables.insert("Carrot")
print(duplicateResult.inserted) // false
print(duplicateResult.memberAfterInsert) // "Carrot"
**ii. update(with:)
: Add or update an element in the set.
let oldValue = vegetables.update(with: "Cucumber")
// If "Cucumber" wasn't present, oldValue is nil
// If "Cucumber" was already in the set, oldValue contains the old value
iii. Adding multiple elements: Insert several values at once.
vegetables.formUnion(["Peas", "Corn", "Cucumber"])
// vegetables now contains all elements (with duplicates removed)
B. Removing Elements
**i. remove(_:)
: Remove a specific element from the set.
let removed = vegetables.remove("Broccoli") // Returns "Broccoli"
// vegetables is now {"Carrot", "Spinach", "Peas", "Corn", "Cucumber"}
// Trying to remove a non-existent element
let notRemoved = vegetables.remove("Potato") // Returns nil
**ii. removeFirst()
: Remove an arbitrary element from the set.
if !vegetables.isEmpty {
let removedItem = vegetables.removeFirst()
print("Removed: \(removedItem)")
}
**iii. removeAll()
: Clear the entire set.
vegetables.removeAll()
// vegetables is now an empty set
// You can keep capacity allocated for performance reasons:
vegetables.removeAll(keepingCapacity: true)
C. Updating Elements
i. Replacing elements: Since sets enforce uniqueness, you must remove and add to replace.
var fruitVarieties: Set<String> = ["Gala Apple", "Green Grape", "Navel Orange"]
// To "update" an element, remove the old one and insert the new one
fruitVarieties.remove("Gala Apple")
fruitVarieties.insert("Honeycrisp Apple")
ii. Updating through set operations: Replace parts of the set based on conditions.
// Remove all elements containing "Green" and add new elements
let elementsToRemove = fruitVarieties.filter { $0.contains("Green") }
fruitVarieties.subtract(elementsToRemove)
fruitVarieties.formUnion(["Red Grape", "Black Grape"])
5. Set Operations and Methods
A. Set Membership and Equality
**i. contains(_:)
: Test whether a set contains a specific element.
let vowels: Set<Character> = ["a", "e", "i", "o", "u"]
let containsA = vowels.contains("a") // true
let containsX = vowels.contains("x") // false
**ii. isEmpty
: Check if a set has no elements.
let empty: Set<Int> = []
print(empty.isEmpty) // true
print(vowels.isEmpty) // false
iii. Equality and subset operations: Compare sets.
let setA: Set<Int> = [1, 2, 3]
let setB: Set<Int> = [1, 2, 3]
let setC: Set<Int> = [1, 2, 3, 4]
print(setA == setB) // true
print(setA == setC) // false
// Check if a set is a subset of another
let isSubset = setA.isSubset(of: setC) // true
let isSuperset = setC.isSuperset(of: setA) // true
// Strict subset/superset (not equal)
let isStrictSubset = setA.isStrictSubset(of: setC) // true
let isStrictSuperset = setC.isStrictSuperset(of: setA) // true
B. Mathematical Set Operations
**i. union(_:)
: Create a new set with all elements from both sets.
let primeNumbers: Set<Int> = [2, 3, 5, 7]
let oddNumbers: Set<Int> = [1, 3, 5, 7, 9]
let unionSet = primeNumbers.union(oddNumbers) // {1, 2, 3, 5, 7, 9}
**ii. intersection(_:)
: Create a new set with only elements common to both sets.
let intersectionSet = primeNumbers.intersection(oddNumbers) // {3, 5, 7}
**iii. subtracting(_:)
: Create a new set with elements not in the second set.
let primeButNotOdd = primeNumbers.subtracting(oddNumbers) // {2}
let oddButNotPrime = oddNumbers.subtracting(primeNumbers) // {1, 9}
**iv. symmetricDifference(_:)
: Create a new set with elements in either set but not both.
let symmetricDiff = primeNumbers.symmetricDifference(oddNumbers) // {1, 2, 9}
C. Mutating Set Operations
**i. formUnion(_:)
: Add all elements from another set to this set.
var numbers: Set<Int> = [1, 2, 3]
numbers.formUnion([3, 4, 5])
// numbers is now {1, 2, 3, 4, 5}
**ii. formIntersection(_:)
: Remove elements that aren't in both sets.
var commonNumbers: Set<Int> = [1, 2, 3, 4]
commonNumbers.formIntersection([3, 4, 5, 6])
// commonNumbers is now {3, 4}
**iii. subtract(_:)
: Remove elements that are in the other set.
var originalSet: Set<Int> = [1, 2, 3, 4, 5]
originalSet.subtract([3, 5, 7])
// originalSet is now {1, 2, 4}
**iv. formSymmetricDifference(_:)
: Keep only elements that are in one set but not both.
var symmetricSet: Set<Int> = [1, 2, 3]
symmetricSet.formSymmetricDifference([2, 3, 4])
// symmetricSet is now {1, 4}
6. Set Iteration
A. Basic Iteration
i. Iterating through all elements: Standard for-in loops.
let colors: Set<String> = ["Red", "Green", "Blue"]
for color in colors {
print(color)
}
// Note: Order is not guaranteed
ii. Sorted iteration: Iterate through elements in a specific order.
// Iterate in ascending order
for color in colors.sorted() {
print(color) // "Blue", "Green", "Red" (alphabetical order)
}
// Custom sorting
for color in colors.sorted(by: { $0.count < $1.count }) {
print(color) // Sorts by string length
}
B. Functional Iteration
i. Using higher-order functions: Applying operations to all elements.
let numbers: Set<Int> = [1, 2, 3, 4, 5]
// Using forEach
numbers.forEach { number in
print("\(number) squared is \(number * number)")
}
// Transforming with map (returns an array, not a set)
let doubled = numbers.map { $0 * 2 } // [2, 4, 6, 8, 10]
// Filtering
let evenNumbers = numbers.filter { $0 % 2 == 0 } // [2, 4]
// Reducing
let sum = numbers.reduce(0, +) // 15
ii. Chaining operations: Combine multiple operations for concise code.
let result = numbers
.filter { $0 % 2 == 1 } // Keep odd numbers
.map { $0 * $0 } // Square them
.reduce(0, +) // Sum the squares
print(result) // 35 (1² + 3² + 5²)
7. Common Set Patterns and Use Cases
A. Removing Duplicates from Arrays
i. Converting arrays to sets and back: The quickest way to ensure uniqueness.
let duplicateNames = ["Alice", "Bob", "Alice", "Charlie", "Bob"]
let uniqueNames = Array(Set(duplicateNames)) // Creates a new array with unique elements
// Note: This loses the original order
// To maintain order while removing duplicates:
let orderedUniqueNames = NSOrderedSet(array: duplicateNames).array as! [String]
// Or in pure Swift:
var seen = Set<String>()
let orderedUnique = duplicateNames.filter { seen.insert($0).inserted }
B. Fast Lookups
i. Using sets for efficient membership testing: Much faster than arrays for lookups.
let dictionary = ["apple", "banana", "orange", "grape", "kiwi", "mango"]
let dictionarySet = Set(dictionary)
func spellCheck(_ word: String) -> Bool {
// Using a set is much faster for large dictionaries
return dictionarySet.contains(word.lowercased())
}
spellCheck("apple") // true
spellCheck("pear") // false
ii. Caching with sets: Store computed values for quick reuse.
var computedValues = Set<Int>()
func expensiveComputation(for value: Int) -> Int {
// If we've already computed this value, don't do it again
if computedValues.contains(value) {
print("Using cached value")
return value * 2 // Simplified example
}
print("Computing new value")
// Pretend this is an expensive operation
let result = value * 2
computedValues.insert(value)
return result
}
C. Set-based Algorithms
i. Finding common elements: Use set operations for efficient comparisons.
let alicesFavorites: Set<String> = ["Pizza", "Chocolate", "Sushi"]
let bobsFavorites: Set<String> = ["Burger", "Chocolate", "Pizza"]
// Foods they both like
let commonFavorites = alicesFavorites.intersection(bobsFavorites)
// Foods either of them likes
let allFavorites = alicesFavorites.union(bobsFavorites)
// Foods only Alice likes
let onlyAlices = alicesFavorites.subtracting(bobsFavorites)
// Foods only one of them likes
let uniqueFavorites = alicesFavorites.symmetricDifference(bobsFavorites)
ii. Implementing filters and blocklists: Quick verification against known values.
let blockedWords: Set<String> = ["spam", "free", "offer", "limited"]
func isSpam(_ message: String) -> Bool {
let words = message.lowercased().split(separator: " ").map(String.init)
return !Set(words).intersection(blockedWords).isEmpty
}
isSpam("Check out this free offer") // true
isSpam("Hello, how are you?") // false
8. Sets in SwiftUI
A. Using Sets for Data Models
i. Managing unique selections: When you need to maintain a collection of unique items.
struct MultiSelectionView: View {
@State private var selectedItems: Set<String> = []
let allItems = ["Option 1", "Option 2", "Option 3", "Option 4"]
var body: some View {
List {
ForEach(allItems, id: \.self) { item in
Button(action: {
// Toggle selection (using a Set makes this simple)
if selectedItems.contains(item) {
selectedItems.remove(item)
} else {
selectedItems.insert(item)
}
}) {
HStack {
Text(item)
Spacer()
if selectedItems.contains(item) {
Image(systemName: "checkmark")
}
}
}
}
}
.navigationTitle("Select Items")
}
}
ii. Tracking states: Using sets to monitor which items are in particular states.
struct TaskListView: View {
@State private var tasks = ["Task 1", "Task 2", "Task 3", "Task 4"]
@State private var completedTasks: Set<String> = []
var body: some View {
List {
ForEach(tasks, id: \.self) { task in
Button(action: {
// Toggle completion
if completedTasks.contains(task) {
completedTasks.remove(task)
} else {
completedTasks.insert(task)
}
}) {
HStack {
Text(task)
Spacer()
if completedTasks.contains(task) {
Image(systemName: "checkmark.circle.fill")
.foregroundColor(.green)
} else {
Image(systemName: "circle")
.foregroundColor(.gray)
}
}
}
}
}
.navigationTitle("Task List")
}
}
B. Filtering Data with Sets
i. Implementing tag-based filtering: Using sets to filter content by multiple tags.
struct ContentItem: Identifiable, Hashable {
let id = UUID()
let title: String
let tags: Set<String>
}
struct FilteredContentView: View {
let allItems = [
ContentItem(title: "SwiftUI Tutorial", tags: ["iOS", "SwiftUI", "Tutorial"]),
ContentItem(title: "Networking in Swift", tags: ["iOS", "Networking", "Tutorial"]),
ContentItem(title: "UIKit Tips", tags: ["iOS", "UIKit", "Tips"])
]
@State private var selectedTags: Set<String> = []
var filteredItems: [ContentItem] {
if selectedTags.isEmpty {
return allItems
}
return allItems.filter { !$0.tags.intersection(selectedTags).isEmpty }
}
var allTags: [String] {
// Get all unique tags
return Array(Set(allItems.flatMap { $0.tags })).sorted()
}
var body: some View {
VStack {
// Tag selection
ScrollView(.horizontal, showsIndicators: false) {
HStack {
ForEach(allTags, id: \.self) { tag in
Button(action: {
if selectedTags.contains(tag) {
selectedTags.remove(tag)
} else {
selectedTags.insert(tag)
}
}) {
Text(tag)
.padding(.horizontal, 10)
.padding(.vertical, 5)
.background(selectedTags.contains(tag) ? Color.blue : Color.gray.opacity(0.3))
.foregroundColor(selectedTags.contains(tag) ? .white : .primary)
.cornerRadius(15)
}
}
}
.padding()
}
// Filtered content
List(filteredItems) { item in
VStack(alignment: .leading) {
Text(item.title)
.font(.headline)
HStack {
ForEach(Array(item.tags), id: \.self) { tag in
Text(tag)
.font(.caption)
.padding(.horizontal, 8)
.padding(.vertical, 4)
.background(Color.gray.opacity(0.2))
.cornerRadius(8)
}
}
}
}
}
.navigationTitle("Content Library")
}
}
9. Best Practices and Tips
A. Safety Considerations
i. Hashable requirement: All elements in a Set must conform to the Hashable protocol.
// Basic types like Int, String, and Double already conform to Hashable
// For custom types, you need to conform to Hashable
struct User: Hashable {
let id: Int
let name: String
// If your type has complex properties, you may need to implement
// the hash(into:) function manually
func hash(into hasher: inout Hasher) {
hasher.combine(id)
// We only use id for hashing to ensure uniqueness
}
// If you're manually implementing hash(into:), you should also
// implement the == operator
static func == (lhs: User, rhs: User) -> Bool {
return lhs.id == rhs.id
}
}
ii. Mutability considerations: Like arrays, sets must be declared with var
to be mutable.
let immutableSet: Set<Int> = [1, 2, 3]
// immutableSet.insert(4) // Error: Cannot use mutating member on immutable value
var mutableSet: Set<Int> = [1, 2, 3]
mutableSet.insert(4) // Works fine
iii. Set operations and nil handling: Be cautious with methods that might return nil.
let numbers: Set<Int> = [1, 2, 3]
// Safely remove elements
if let removed = numbers.remove(2) {
print("Removed \(removed)")
} else {
print("Element was not in the set")
}
// Safely access elements
if let anyNumber = numbers.first {
print("Got \(anyNumber)")
} else {
print("Set is empty")
}
B. Performance Tips
i. Choose sets over arrays for membership testing: Sets provide O(1) lookups compared to O(n) for arrays.
let largeArray = Array(1...10000)
let largeSet = Set(largeArray)
// Inefficient for large collections
func isInArray(_ value: Int, in array: [Int]) -> Bool {
return array.contains(value) // O(n) operation
}
// Much more efficient
func isInSet(_ value: Int, in set: Set<Int>) -> Bool {
return set.contains(value) // O(1) operation
}
// For repeated lookups, convert to a set first
func findValuesInArray(_ values: [Int], in array: [Int]) -> [Int] {
let setRepresentation = Set(array) // Convert once
return values.filter { setRepresentation.contains($0) }
}
ii. Set capacity management: Pre-allocate for better performance with large sets.
var largeSet = Set<Int>()
largeSet.reserveCapacity(10000) // Allocate space for 10,000 elements
for i in 1...10000 {
largeSet.insert(i)
}
iii. Optimizing set operations: Choose the right operation for the task.
let set1: Set<Int> = [1, 2, 3, 4, 5]
let set2: Set<Int> = [4, 5, 6, 7, 8]
// Less efficient approach for checking common elements
func hasCommonElements_inefficient(_ a: Set<Int>, _ b: Set<Int>) -> Bool {
for element in a {
if b.contains(element) {
return true
}
}
return false
}
// More efficient approach
func hasCommonElements_efficient(_ a: Set<Int>, _ b: Set<Int>) -> Bool {
return !a.intersection(b).isEmpty
}
// For operations on many sets, consider combining operations
func commonElementsInAllSets(_ sets: [Set<Int>]) -> Set<Int> {
guard let first = sets.first else { return [] }
return sets.dropFirst().reduce(first) { $0.intersection($1) }
}
iv. Memory usage: Be aware of the memory implications of set operations.
let largeSet1 = Set(1...1000000)
let largeSet2 = Set(500000...1500000)
// This creates a new set and might use significant memory
let union = largeSet1.union(largeSet2)
// To avoid excessive memory usage with large sets,
// consider using the in-place variants when possible
var mutableLarge = Set(1...1000000)
mutableLarge.formUnion(500000...1500000)
Conclusion
Sets are a powerful and often underutilized collection type in Swift. They excel at tasks requiring unique elements and fast lookups, making them perfect for membership testing, removing duplicates, and performing mathematical set operations.
Unlike arrays, sets don't maintain a specific order and automatically enforce uniqueness, which simplifies many common programming tasks. Their hash-based implementation provides exceptional performance for operations like insertion, deletion, and membership testing.
By mastering sets, you'll add an essential tool to your Swift programming toolkit that will help you write more efficient and expressive code. Whether you're filtering data, managing selections in a UI, or solving complex algorithmic problems, sets are often the right data structure for the job.
Learn with videos and source files. Available to Pro subscribers only.
Purchase includes access to 50+ courses, 320+ premium tutorials, 300+ hours of videos, source files and certificates.
Templates and source code
Download source files
Download the videos and assets to refer and learn offline without interuption.
Design template
Source code for all sections
Video files, ePub and subtitles
Videos
Assets
1
Building Your iOS Development Foundation
Master the fundamentals of Swift programming with hands-on examples designed for beginners and experienced developers alike
2
SwiftUI Print Debugging
Print debugging: Unlock the invisible processes with strategic print statements that illuminate state changes, view lifecycles and data flow
18:04
3
Comments Documentation Waypoints
Transform your code from mysterious instructions to a comprehensive narrative with strategic comments that explain the why
22:02
4
Variables and Constants
Learn when and how to use variables and constants to write safer, more efficient SwiftUI code
11:37
5
Strings and Interpolation
Learn essential string operations in Swift: Build better iOS apps with efficient text handling techniques
13:22
6
Swift Operators: The Foundation of SwiftUI Logic
Building powerful iOS apps through the language of operations
5:34
7
Swift Unary Operators
Mastering the elegant simplicity of unary operators for cleaner, more expressive SwiftUI code that transforms your UI with minimal syntax
15:00
8
Swift Binary Operators
Master the two-operand symbols that transform complex interface logic into concise, readable declarations
3:36
9
Arithmetic Operators
Learn how to implement and optimize arithmetic operations in SwiftUI, from basic calculations to complex mathematical interfaces
6:11
10
If-Else and Comparison Operators
Building Dynamic SwiftUI: Mastering If-Else and Comparison Operators
12:32
11
Logical Operators
Master SwiftUI's logical operators: Building intelligent iOS apps with robust decision-making systems
6:03
12
Ternary Operators
Use the power of Swift's ternary conditional operator to create dynamic, responsive interfaces with minimal code in SwiftUI
6:22
13
Blocks and Scope
A comprehensive guide to writing clean, organized code through proper variable management and state control
10:22
14
Swift Collections: Arrays, Sets, and Dictionaries Overview
Organize Your Data Effectively: Learn How to Choose and Optimize the Perfect Collection Type for Your SwiftUI Applications
7:00
15
Swift Arrays
Organize, transform, and display your data with Swift's most essential collection structure
8:54
16
Swift Sets
Mastering Unique Collections with Fast Lookups and Powerful Operations
11:03
17
Swift Dictionaries
Master Swift's key-value collection type with practical examples for efficient data storage, retrieval, and transformation in SwiftUI apps
18
For-Loops and Range
For-Loops and Ranges: Learn how to repeat code efficiently and work with sequences of numbers in Swift
19
Optionals
Optionals in Swift: Understanding Swift's Safety System for Handling Missing Values
20
Functions
Functions in Swift: Building Reusable Code Blocks to Organize and Streamline Your App
Meet the instructor
We all try to be consistent with our way of teaching step-by-step, providing source files and prioritizing design in our courses.
Sourasith Phomhome
UI Designer
Designer at Design+Code
20 courses - 78 hours
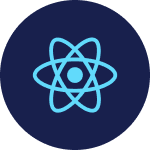
Master Agentic Workflows
In this course, you’ll learn how to add agents to your workflows. An agent workflow is more than just a simple automation. Instead of following a fixed script, agents can make decisions, adjust to changes, and figure out the best way to complete a task. We’ll start by exploring what MCP servers are and all the new possibilities they bring. Then, we’ll dive into agentic frameworks that make it easy to build flexible, helpful agents that can take care of your everyday tasks.
2 hrs
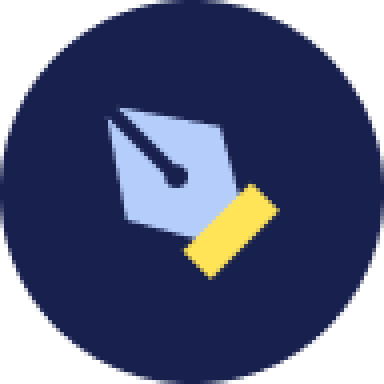
Design Multiple Apps with Figma and AI
In this course, you’ll learn to design multiple apps using Figma and AI-powered tools, tackling a variety of real-world UI challenges. Each week, a new episode will guide you through a different design, helping you master essential UI/UX principles and workflows
4 hrs
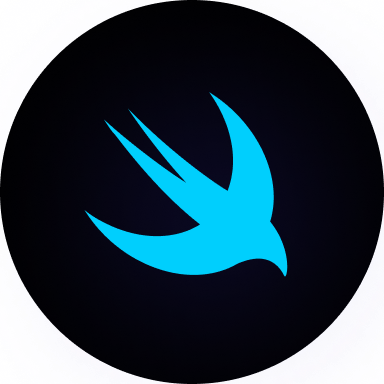
SwiftUI Fundamentals Handbook
A comprehensive guide to mastering Swift programming fundamentals, designed for aspiring iOS developers. This handbook provides a structured approach to learning Swift's core concepts, from basic syntax to advanced programming patterns. Through carefully sequenced chapters, readers will progress from essential programming concepts to object-oriented principles, building a solid foundation for SwiftUI development. Each topic includes practical examples and clear explanations, ensuring a thorough understanding of Swift's capabilities. This handbook serves as both a learning resource and a reference guide, covering fundamental concepts required for modern iOS development. Topics are presented in a logical progression, allowing readers to build their knowledge systematically while gaining practical programming skills.
2 hrs
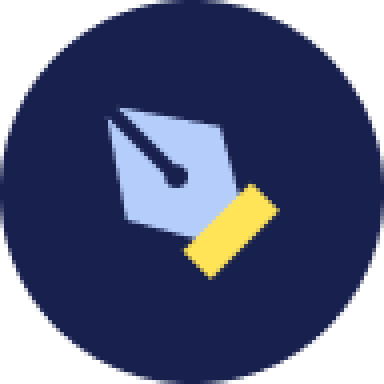
Design and Code User Interfaces with Galileo and Claude AI
In this course, you’ll learn how to use AI tools to make UI/UX design faster and more efficient. We’ll start with Galileo AI to create basic designs, providing a solid foundation for your ideas. Next, we’ll refine these designs in Figma to match your personal style, and finally, we’ll use Claude AI to turn them into working code—eliminating the need for traditional prototyping.
4 hrs
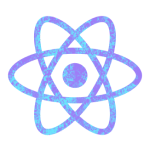
Build a React Native app with Claude AI
This comprehensive course explores the integration of cutting-edge AI tools into the React Native development workflow, revolutionizing the approach to mobile application creation. Participants will learn to leverage AI-powered platforms such as Claude and Locofy to expedite coding processes, enhance problem-solving capabilities, and optimize productivity.
14 hrs
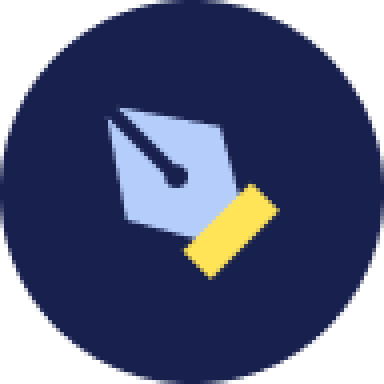
Design and Prototype for iOS 18
Design and Prototype for iOS 18 is an immersive course that equips you with the skills to create stunning, user-friendly mobile applications. From mastering Figma to understanding iOS 18's latest design principles, you'll learn to craft two real-world apps - a Car Control interface and an AI assistant.
3 hrs
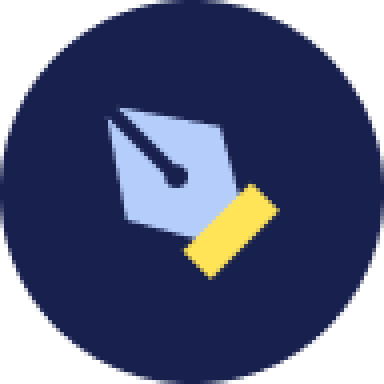
Master Responsive Layouts in Figma
Creating responsive layouts is a must-have skill for any UI/UX designer. With so many different devices and screen sizes, designing interfaces that look great and work well on all platforms is necessary. Mastering this skill will make you stand out in the field. In this course, we'll start from scratch to create this beautiful design using Figma. You'll learn how to make layouts that are easy to use and work well on any device. We'll cover key concepts and tools to help you master responsive design in Figma.
2 hrs
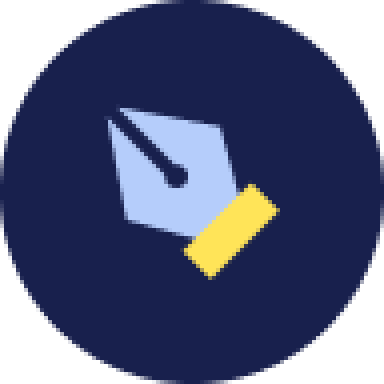
UI UX Design with Mobbin and Figma
Mobbin is a powerful tool for UI/UX designers seeking inspiration and innovative design solutions. This platform offers a vast collection of real-world mobile app designs, providing a treasure trove of UI elements and layouts.
2 hrs
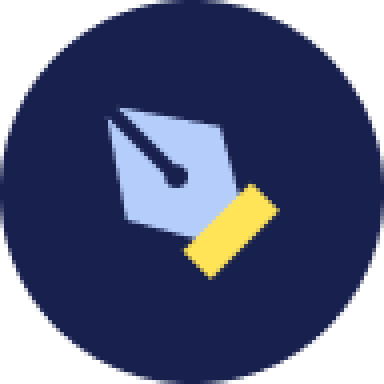
3D UI Interactive Web Design with Spline
Learn to create 3D designs and UI interactions such as 3D icons, UI animations, components, variables, screen resize, scrolling interactions, as well as exporting, optimizing, and publishing your 3D assets on websites
3 hrs
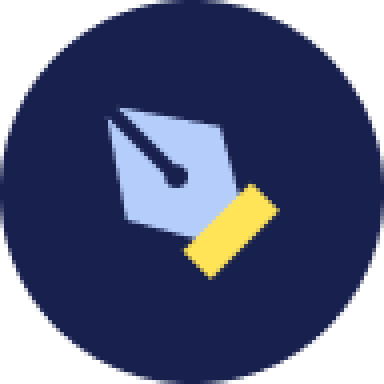
Design and Prototype for iOS 17 in Figma
Crafting engaging experiences for iOS 17 and visionOS using the Figma design tool. Learn about Figma's new prototyping features, Dev Mode, variables and auto layout.
6 hrs
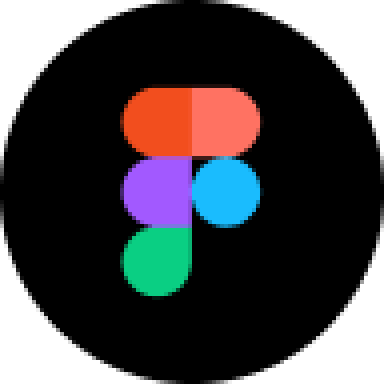
Design and Prototype Apps with Midjourney
A comprehensive course on transforming Midjourney concepts into interactive prototypes using essential design techniques and AI tools
8 hrs
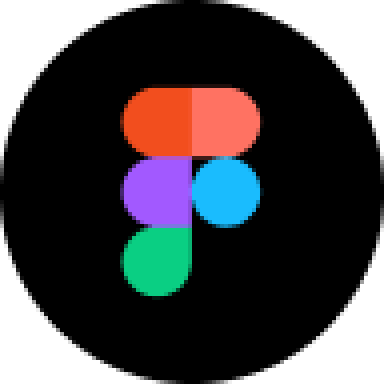
iOS Design with Midjourney and Figma
Learn the fundamentals of App UI design and master the art of creating beautiful and intuitive user interfaces for mobile applications
1 hrs
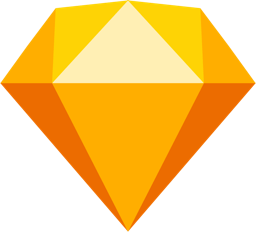
UI Design for iOS, Android and Web in Sketch
Create a UI design from scratch using Smart Layout, Components, Prototyping in Sketch app
1 hrs
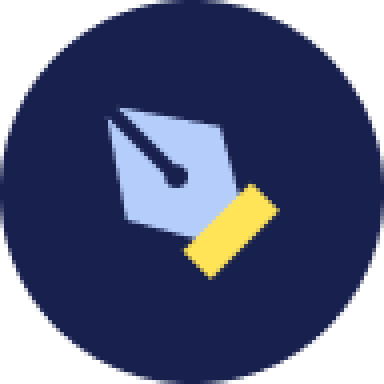
UI Design a Camera App in Figma
Design a dark, vibrant and curvy app design from scratch in Figma. Design glass icons, lens strokes and realistic buttons.
1 hrs
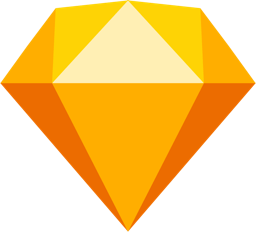
UI Design for iOS 16 in Sketch
A complete guide to designing for iOS 16 with videos, examples and design files
3 hrs
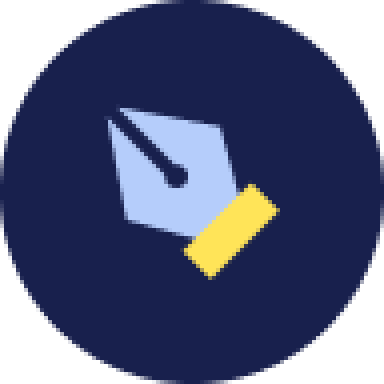
Prototyping in Figma
Learn the basics of prototyping in Figma by creating interactive flows from custom designs
1 hrs
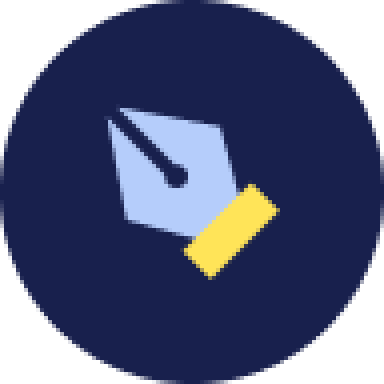
UI Design Quick Websites in Figma
Learn how to design a portfolio web UI from scratch in Figma
1 hrs
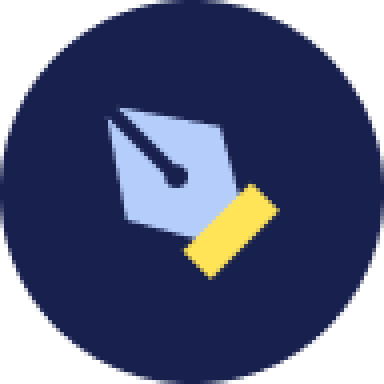
UI Design Android Apps in Figma
Design Android application UIs from scratch using various tricks and techniques in Figma
2 hrs
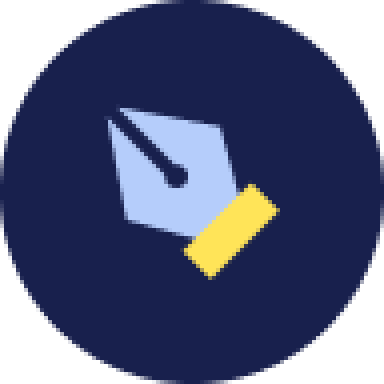
UI Design Quick Apps in Figma
Design application UIs from scratch using various tricks and techniques in Figma
12 hrs
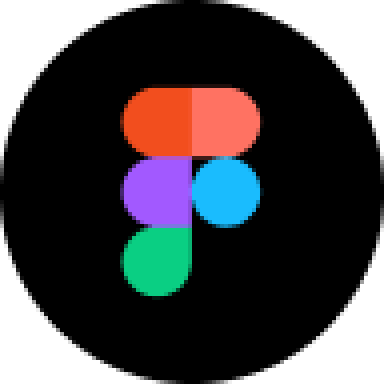
Figma Handbook
A comprehensive guide to the best tips and tricks in Figma
6 hrs