Swift Arrays: Best Practices in SwiftUI Part 2
Add to favorites
Integrate arrays with SwiftUI, optimize performance, and implement professional-grade patterns for production apps
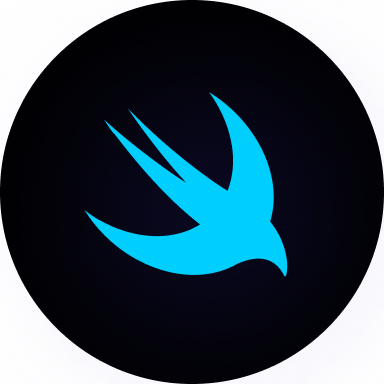
SwiftUI Fundamentals Handbook
1
Building Your iOS Development Foundation
2
Print Statements Debugging
18:04
3
Comments: Documentation Waypoints in Your SwiftUI Codebase
14:39
4
Variables and Constants
11:37
5
Strings and Interpolation
13:22
6
Swift Operators: The Foundation of SwiftUI Logic
7
Unary Operators
8
Binary Operators
9
Arithmetic Operators
10
If-Else and Comparison Operators
11
Logical Operators
12
Ternary Operators
13
Blocks and Scope
10:22
14
Swift Collections: Arrays, Sets, and Dictionaries Overview
15
Swift Arrays: The Basics Part 1
16
Swift Arrays: Best Practices in SwiftUI Part 2
4. Arrays in SwiftUI
Open the "Arrays" Xcode project to follow along with hands-on examples. This project contains pre-configured SwiftUI views demonstrating each concept, allowing you to experiment with the code and see immediate results in the preview canvas.
The integration between arrays and SwiftUI represents one of the most powerful aspects of modern iOS development. While the previous sections focused on how arrays work in Swift generally, this section explores their specific role in SwiftUI's declarative interface paradigm. Understanding this relationship is crucial because arrays often serve as the backbone of dynamic user interfaces, powering everything from simple lists to complex, data-driven layouts. The techniques covered here will help you create responsive, efficient UIs that automatically update when your underlying data changes, one of the key advantages of SwiftUI's reactive approach to interface development.
A. Displaying Arrays in UI
i. ForEach and List views - SwiftUI provides two primary components for rendering collections of data: ForEach
and List
. The ForEach
view is a structural component that generates views dynamically based on a collection, allowing you to create repetitive interface elements without manually duplicating code. It can be used within any container view, making it incredibly versatile for grid layouts, stacks, or custom arrangements. The List
view, by contrast, is a specialized container that combines ForEach
with scrolling behavior, navigation capabilities, and built-in styling to create familiar list-based interfaces. Together, these components form the foundation of collection rendering in SwiftUI, enabling everything from simple item listings to complex data presentations with minimal code.
// Basic ForEach example inside a VStack
struct BasicForEachDemo: View {
let colors = ["Red", "Green", "Blue", "Yellow", "Purple"]
var body: some View {
VStack(alignment: .leading, spacing: 10) {
Text("Available Colors")
.font(.headline)
ForEach(colors, id: \.self) { color in
Text(color)
.foregroundColor(.white)
.padding(8)
.background(Color(color.lowercased()))
.cornerRadius(4)
}
}
.padding()
}
}
// List example with sections
struct ListDemo: View {
let fruits = ["Apple", "Banana", "Cherry", "Mango"]
let vegetables = ["Carrot", "Broccoli", "Spinach"]
var body: some View {
List {
Section(header: Text("Fruits")) {
ForEach(fruits, id: \.self) { fruit in
Text(fruit)
}
}
Section(header: Text("Vegetables")) {
ForEach(vegetables, id: \.self) { vegetable in
Text(vegetable)
}
}
}
.listStyle(GroupedListStyle())
}
}
// Dynamic content with conditional rendering
struct DynamicListDemo: View {
let items = ["Item 1", "Item 2", "Item 3", "Item 4", "Item 5"]
@State private var showExtendedList = false
var body: some View {
VStack {
List {
ForEach(items.prefix(showExtendedList ? items.count : 3), id: \.self) { item in
Text(item)
}
}
Button(showExtendedList ? "Show Less" : "Show More") {
withAnimation {
showExtendedList.toggle()
}
}
.padding()
}
}
}
// Combining ForEach with other layout containers
struct GridLayoutDemo: View {
let colors = ["Red", "Green", "Blue", "Yellow", "Purple", "Orange", "Pink", "Cyan"]
let columns = [GridItem(.adaptive(minimum: 100))]
var body: some View {
ScrollView {
LazyVGrid(columns: columns, spacing: 20) {
ForEach(colors, id: \.self) { color in
RoundedRectangle(cornerRadius: 10)
.fill(Color(color.lowercased()))
.frame(height: 100)
.overlay(
Text(color)
.foregroundColor(.white)
.fontWeight(.bold)
)
}
}
.padding()
}
}
}
ii. Identifiable protocol - SwiftUI's collection views need a way to uniquely identify each element to efficiently track changes and update only the necessary parts of the interface. The Identifiable
protocol formalizes this requirement by establishing a standard way for types to provide unique identifiers. When your models conform to Identifiable
, SwiftUI can automatically detect and respond to changes in your data, enabling smooth animations and preventing performance issues like view flickering or state loss during updates. This protocol simplifies your view code by eliminating the need for explicit ID parameters and makes your data models more reusable across different SwiftUI views. For complex applications with nested data structures, this approach becomes increasingly valuable as it encourages consistent identification patterns throughout your model layer.
// Basic Identifiable implementation
struct Task: Identifiable {
let id = UUID() // Automatic unique identifier
var title: String
var isCompleted: Bool
}
// Using Identifiable models with ForEach and List
struct TaskListView: View {
let tasks = [
Task(title: "Learn SwiftUI", isCompleted: true),
Task(title: "Build a demo app", isCompleted: false),
Task(title: "Submit to App Store", isCompleted: false)
]
var body: some View {
List(tasks) { task in
HStack {
Text(task.title)
Spacer()
if task.isCompleted {
Image(systemName: "checkmark.circle.fill")
.foregroundColor(.green)
}
}
}
// Notice we don't need to specify 'id: \.id' because Task is Identifiable
}
}
// Using custom ID properties with Identifiable
struct Product: Identifiable {
let id: String // Custom ID (e.g., from a database)
let name: String
let price: Double
init(id: String, name: String, price: Double) {
self.id = id
self.name = name
self.price = price
}
}
// More complex example with nested Identifiable types
struct Department: Identifiable {
let id = UUID()
let name: String
let employees: [Employee]
}
struct Employee: Identifiable {
let id = UUID()
let name: String
let role: String
}
struct OrganizationView: View {
let departments = [
Department(
name: "Engineering",
employees: [
Employee(name: "Alice", role: "iOS Developer"),
Employee(name: "Bob", role: "Backend Developer")
]
),
Department(
name: "Design",
employees: [
Employee(name: "Charlie", role: "UI Designer"),
Employee(name: "Diana", role: "UX Researcher")
]
)
]
var body: some View {
List {
ForEach(departments) { department in
Section(header: Text(department.name)) {
ForEach(department.employees) { employee in
VStack(alignment: .leading) {
Text(employee.name)
.font(.headline)
Text(employee.role)
.font(.subheadline)
.foregroundColor(.secondary)
}
}
}
}
}
}
}
iii. id parameter - While conforming to the Identifiable
protocol is the preferred approach for model types you control, the id
parameter offers a flexible alternative for working with arrays of types you can't modify or when you need temporary identifiers for simple data structures. By specifying a key path to a property that can uniquely identify each element, you can use virtually any collection with SwiftUI's iteration views. This approach is particularly useful when working with primitive types, third-party libraries, or when you need to use a different identifier in specific contexts. The id
parameter ensures that you're never limited by the types in your arrays, maintaining SwiftUI's flexibility while preserving its need for stable identifiers to track element changes and maintain view state efficiently.
// Using id with simple types
struct SimpleTypeExample: View {
let numbers = [42, 17, 99, 3, 256]
var body: some View {
List {
ForEach(numbers, id: \.self) { number in
Text("Number: \(number)")
}
}
// Using \.self works for types that are Hashable
}
}
// Working with third-party types
struct ThirdPartyModel {
// Imagine this comes from a library you can't modify
let identifier: Int
let title: String
let detail: String
}
struct ThirdPartyListView: View {
let items = [
ThirdPartyModel(identifier: 1, title: "First Item", detail: "Details about first item"),
ThirdPartyModel(identifier: 2, title: "Second Item", detail: "Details about second item"),
ThirdPartyModel(identifier: 3, title: "Third Item", detail: "Details about third item")
]
var body: some View {
List {
ForEach(items, id: \.identifier) { item in
VStack(alignment: .leading) {
Text(item.title)
.font(.headline)
Text(item.detail)
.font(.body)
.foregroundColor(.secondary)
}
}
}
}
}
// Using compound properties as identifiers
struct UserSession {
let username: String
let loginDate: Date
let deviceID: String
}
struct ActiveSessionsView: View {
let sessions = [
UserSession(username: "alice", loginDate: Date(), deviceID: "iPhone12"),
UserSession(username: "alice", loginDate: Date().addingTimeInterval(-3600), deviceID: "MacBook"),
UserSession(username: "bob", loginDate: Date(), deviceID: "iPad")
]
var body: some View {
List {
// Combining multiple properties for a unique identifier
ForEach(sessions, id: \.self) { session in
HStack {
Text(session.username)
Spacer()
Text(session.deviceID)
Spacer()
Text(session.loginDate, style: .time)
}
}
}
}
}
// Making a custom key path identifiable wrapper
struct IdentifiableWrapper<T>: Identifiable {
let id: String
let value: T
init(_ value: T, id: String) {
self.value = value
self.id = id
}
}
struct GenericIdentifiableExample: View {
// For complex scenarios where you need custom identification logic
let data = [
IdentifiableWrapper("Hello", id: "string-1"),
IdentifiableWrapper("World", id: "string-2"),
IdentifiableWrapper("SwiftUI", id: "string-3")
]
var body: some View {
ForEach(data) { wrapper in
Text(wrapper.value)
}
}
}
B. Binding Arrays to State
i. @State and arrays - The @State
property wrapper is fundamental to SwiftUI's reactivity model, automatically triggering view updates when the data it contains changes. When applied to arrays, it enables dynamic interfaces that respond to collection modifications such as adding, removing, or updating elements. However, working with @State
arrays requires understanding Swift's value semantics – array mutations must modify the full array variable to trigger updates properly. This section explores patterns for effectively managing array state, including best practices for array modifications, handling element changes, and structuring code to maintain clean separation between data and UI logic. These techniques are essential for building responsive SwiftUI interfaces that seamlessly reflect your application's changing data state.
// Basic State array management
struct TodoListView: View {
@State private var tasks = [
"Learn Swift fundamentals",
"Study SwiftUI layout system",
"Build a simple app",
"Explore Combine framework"
]
@State private var newTaskText = ""
var body: some View {
VStack {
List {
ForEach(tasks, id: \.self) { task in
Text(task)
}
.onDelete(perform: deleteTasks)
}
HStack {
TextField("New task", text: $newTaskText)
.textFieldStyle(RoundedBorderTextFieldStyle())
Button(action: addTask) {
Image(systemName: "plus.circle.fill")
.foregroundColor(.blue)
}
.disabled(newTaskText.isEmpty)
}
.padding()
}
}
func addTask() {
// Important: Modifying the full array ensures SwiftUI detects the change
tasks.append(newTaskText)
newTaskText = ""
}
func deleteTasks(at offsets: IndexSet) {
tasks.remove(atOffsets: offsets)
}
}
// Working with custom types in State arrays
struct Task {
let id = UUID()
var title: String
var isCompleted: Bool
}
extension Task: Identifiable {}
struct AdvancedTodoListView: View {
@State private var tasks = [
Task(title: "Design user interface", isCompleted: true),
Task(title: "Implement core functionality", isCompleted: false),
Task(title: "Write unit tests", isCompleted: false),
Task(title: "Fix reported bugs", isCompleted: false)
]
@State private var newTaskTitle = ""
var body: some View {
VStack {
List {
ForEach(tasks) { task in
TaskRow(task: task, toggleAction: {
toggleTask(task)
})
}
.onDelete(perform: deleteTasks)
}
HStack {
TextField("New task", text: $newTaskTitle)
.textFieldStyle(RoundedBorderTextFieldStyle())
Button(action: addTask) {
Text("Add")
}
.disabled(newTaskTitle.isEmpty)
}
.padding()
}
}
func addTask() {
let newTask = Task(title: newTaskTitle, isCompleted: false)
tasks.append(newTask)
newTaskTitle = ""
}
func toggleTask(_ task: Task) {
// Finding and updating an element in the array
if let index = tasks.firstIndex(where: { $0.id == task.id }) {
// Create a new task with toggled completion status
var updatedTask = tasks[index]
updatedTask.isCompleted.toggle()
// Replace the old task with the updated one
tasks[index] = updatedTask
}
}
func deleteTasks(at offsets: IndexSet) {
tasks.remove(atOffsets: offsets)
}
}
struct TaskRow: View {
let task: Task
let toggleAction: () -> Void
var body: some View {
HStack {
Button(action: toggleAction) {
Image(systemName: task.isCompleted ? "checkmark.circle.fill" : "circle")
.foregroundColor(task.isCompleted ? .green : .gray)
}
Text(task.title)
.strikethrough(task.isCompleted)
.foregroundColor(task.isCompleted ? .gray : .primary)
}
}
}
ii. @Binding for array elements - The @Binding
property wrapper creates a two-way connection to a value owned by a parent view, allowing child views to modify data without directly owning it. When working with arrays, bindings become particularly powerful as they enable individual elements to be edited without complex index management or state synchronization. However, creating bindings to array elements requires careful consideration of Swift's value semantics to ensure changes propagate correctly. This section explores techniques for safely passing bindings to array elements, including index-based approaches, identifier-based lookups, and wrapper types that facilitate clean component design. Understanding these patterns is crucial for building modular SwiftUI interfaces with reusable components that need to modify items within larger collections.
// Creating bindings to array elements
struct EditableTaskList: View {
@State private var tasks = [
Task(title: "Research user needs", isCompleted: false),
Task(title: "Create wireframes", isCompleted: false),
Task(title: "Design UI components", isCompleted: false)
]
var body: some View {
List {
ForEach(Array(tasks.enumerated()), id: \.element.id) { index, _ in
// Create a binding to each array element
EditableTaskRow(task: $tasks[index])
}
}
}
}
struct EditableTaskRow: View {
@Binding var task: Task
@State private var isEditing = false
@State private var editableTitle = ""
var body: some View {
if isEditing {
HStack {
TextField("Task title", text: $editableTitle)
.textFieldStyle(RoundedBorderTextFieldStyle())
Button("Save") {
task.title = editableTitle
isEditing = false
}
}
} else {
HStack {
Button(action: {
task.isCompleted.toggle()
}) {
Image(systemName: task.isCompleted ? "checkmark.circle.fill" : "circle")
.foregroundColor(task.isCompleted ? .green : .gray)
}
Text(task.title)
.strikethrough(task.isCompleted)
Spacer()
Button(action: {
editableTitle = task.title
isEditing = true
}) {
Image(systemName: "pencil")
.foregroundColor(.blue)
}
}
}
}
}
// Alternative approach with element binding wrapper
struct BindingProvider<Element, Content: View>: View {
@Binding var array: [Element]
let content: (Binding<Element>) -> Content
let index: Int
init(array: Binding<[Element]>, index: Int, @ViewBuilder content: @escaping (Binding<Element>) -> Content) {
self._array = array
self.index = index
self.content = content
}
var body: some View {
content($array[index])
}
}
// Using this helper to create cleaner binding code
struct ModularTaskList: View {
@State private var tasks = [
Task(title: "Create project plan", isCompleted: false),
Task(title: "Develop prototype", isCompleted: false),
Task(title: "Test with users", isCompleted: false)
]
var body: some View {
List {
ForEach(Array(tasks.indices), id: \.self) { index in
BindingProvider(array: $tasks, index: index) { taskBinding in
TaskRowWithBinding(task: taskBinding)
}
}
}
}
}
struct TaskRowWithBinding: View {
@Binding var task: Task
var body: some View {
Toggle(task.title, isOn: $task.isCompleted)
}
}
// Complex example with multi-level bindings
struct Project: Identifiable {
let id = UUID()
var title: String
var tasks: [Task]
}
struct ProjectManagementView: View {
@State private var projects = [
Project(
title: "iOS App",
tasks: [
Task(title: "Design UI", isCompleted: false),
Task(title: "Implement core features", isCompleted: false)
]
),
Project(
title: "Website",
tasks: [
Task(title: "Create mockups", isCompleted: false),
Task(title: "Develop frontend", isCompleted: false)
]
)
]
var body: some View {
List {
ForEach(Array(projects.enumerated()), id: \.element.id) { projectIndex, _ in
Section(header: Text(projects[projectIndex].title)) {
ForEach(Array(projects[projectIndex].tasks.enumerated()), id: \.element.id) { taskIndex, _ in
// Creating a binding to a nested array element
TaskRowWithBinding(task: $projects[projectIndex].tasks[taskIndex])
}
}
}
}
}
}
iii. ObservableObject with published arrays - For more complex data models or when sharing array data across multiple views, SwiftUI's ObservableObject
protocol provides a robust solution by allowing objects to publish changes to their properties. When combined with the @Published
property wrapper, arrays become reactive data sources that can drive updates across your entire view hierarchy. This approach is particularly valuable for larger applications where separation of concerns is important, as it allows you to encapsulate array manipulation logic within dedicated model classes while maintaining SwiftUI's reactive updates. This section explores best practices for designing observable models with array properties, including initialization patterns, update methods, and techniques for handling complex state changes while preserving performance.
// Basic ObservableObject with a published array
class TaskManager: ObservableObject {
@Published var tasks: [Task] = [
Task(title: "Plan project scope", isCompleted: false),
Task(title: "Create timeline", isCompleted: false),
Task(title: "Assign resources", isCompleted: false)
]
func addTask(title: String) {
let newTask = Task(title: title, isCompleted: false)
tasks.append(newTask)
}
func toggleCompletion(for taskID: UUID) {
if let index = tasks.firstIndex(where: { $0.id == taskID }) {
tasks[index].isCompleted.toggle()
}
}
func deleteTask(at indices: IndexSet) {
tasks.remove(atOffsets: indices)
}
}
struct TaskManagerView: View {
@StateObject private var taskManager = TaskManager()
@State private var newTaskTitle = ""
var body: some View {
VStack {
List {
ForEach(taskManager.tasks) { task in
HStack {
Button(action: {
taskManager.toggleCompletion(for: task.id)
}) {
Image(systemName: task.isCompleted ? "checkmark.circle.fill" : "circle")
.foregroundColor(task.isCompleted ? .green : .gray)
}
Text(task.title)
.strikethrough(task.isCompleted)
}
}
.onDelete(perform: taskManager.deleteTask)
}
HStack {
TextField("New task", text: $newTaskTitle)
.textFieldStyle(RoundedBorderTextFieldStyle())
Button("Add") {
if !newTaskTitle.isEmpty {
taskManager.addTask(title: newTaskTitle)
newTaskTitle = ""
}
}
}
.padding()
}
}
}
// More complex example with multiple published collections
class ProjectCoordinator: ObservableObject {
@Published var activeProjects: [Project] = []
@Published var archivedProjects: [Project] = []
@Published var team: [Employee] = []
init() {
// Load initial data
activeProjects = [
Project(title: "Mobile App Redesign", tasks: [
Task(title: "User research", isCompleted: true),
Task(title: "Create wireframes", isCompleted: false)
]),
Project(title: "Backend API", tasks: [
Task(title: "Define endpoints", isCompleted: false),
Task(title: "Set up database", isCompleted: false)
])
]
team = [
Employee(name: "Alice Smith", role: "Designer"),
Employee(name: "Bob Johnson", role: "Developer"),
Employee(name: "Carol Williams", role: "Project Manager")
]
}
func addProject(title: String) {
let newProject = Project(title: title, tasks: [])
activeProjects.append(newProject)
}
func archiveProject(id: UUID) {
guard let index = activeProjects.firstIndex(where: { $0.id == id }) else {
return
}
let project = activeProjects.remove(at: index)
archivedProjects.append(project)
}
func addTask(to projectID: UUID, title: String) {
guard let projectIndex = activeProjects.firstIndex(where: { $0.id == projectID }) else {
return
}
let newTask = Task(title: title, isCompleted: false)
activeProjects[projectIndex].tasks.append(newTask)
}
}
// Using multiple views with shared ObservableObject
struct ProjectDashboardView: View {
@StateObject private var coordinator = ProjectCoordinator()
var body: some View {
TabView {
NavigationView {
ActiveProjectsView()
.navigationTitle("Active Projects")
}
.tabItem {
Label("Projects", systemImage: "folder")
}
.environmentObject(coordinator)
NavigationView {
TeamView()
.navigationTitle("Team")
}
.tabItem {
Label("Team", systemImage: "person.3")
}
.environmentObject(coordinator)
}
}
}
struct ActiveProjectsView: View {
@EnvironmentObject var coordinator: ProjectCoordinator
@State private var newProjectTitle = ""
@State private var selectedProject: Project?
var body: some View {
List {
Section(header: Text("Add New Project")) {
HStack {
TextField("Project title", text: $newProjectTitle)
Button("Add") {
if !newProjectTitle.isEmpty {
coordinator.addProject(title: newProjectTitle)
newProjectTitle = ""
}
}
}
}
Section(header: Text("Current Projects")) {
ForEach(coordinator.activeProjects) { project in
NavigationLink(destination: ProjectDetailView(projectID: project.id)) {
VStack(alignment: .leading) {
Text(project.title)
.font(.headline)
Text("\(project.tasks.count) tasks")
.font(.caption)
.foregroundColor(.secondary)
}
}
}
}
}
}
}
struct ProjectDetailView: View {
@EnvironmentObject var coordinator: ProjectCoordinator
let projectID: UUID
@State private var newTaskTitle = ""
private var project: Project? {
coordinator.activeProjects.first { $0.id == projectID }
}
var body: some View {
VStack {
if let project = project {
List {
Section(header: Text("Tasks")) {
ForEach(project.tasks) { task in
Text(task.title)
}
HStack {
TextField("New task", text: $newTaskTitle)
Button("Add") {
if !newTaskTitle.isEmpty {
coordinator.addTask(to: projectID, title: newTaskTitle)
newTaskTitle = ""
}
}
}
}
}
.navigationTitle(project.title)
.toolbar {
ToolbarItem(placement: .navigationBarTrailing) {
Button("Archive") {
coordinator.archiveProject(id: projectID)
}
}
}
} else {
Text("Project not found")
}
}
}
}
struct TeamView: View {
@EnvironmentObject var coordinator: ProjectCoordinator
var body: some View {
List(coordinator.team) { employee in
VStack(alignment: .leading) {
Text(employee.name)
.font(.headline)
Text(employee.role)
.foregroundColor(.secondary)
}
}
}
}
The integration of arrays with SwiftUI's state management system is what transforms static layouts into dynamic, interactive applications. By understanding the different approaches – from simple @State
arrays for local view data, to @Binding
for component communication, to ObservableObject
for complex application models – you gain the ability to structure your code according to the specific needs of your project. Each technique has its place in the SwiftUI developer's toolkit, allowing you to balance simplicity with scalability as your applications grow in complexity. The reactive nature of these state management approaches ensures that your interfaces stay in sync with your data model, one of the core strengths of SwiftUI's declarative paradigm.
5. Array Performance and Best Practices
Understanding how arrays perform in Swift is essential for building responsive, efficient iOS applications. While Swift abstracts away many low-level details, knowing how arrays work under the hood helps you make better decisions when organizing your data and designing your SwiftUI interfaces. This section explores practical performance considerations and common challenges you'll encounter when working with arrays in your day-to-day development.
A. Performance Considerations
i. Understanding array efficiency - How different operations affect performance and when they might slow down your app
Swift arrays are optimized for different kinds of operations, and knowing which operations are fast or slow can significantly impact your app's performance. Adding elements to the end of an array using append()
is extremely efficient because Swift reserves extra space at the end of arrays in anticipation of growth. This means that most append operations happen in constant time (O(1) in computer science terminology).
In contrast, inserting elements at the beginning or middle of an array using insert(at:)
requires Swift to shift all the subsequent elements to make room. For small arrays, this difference is negligible, but for arrays with thousands of elements, it can create noticeable slowdowns. Consider this example:
var smallArray = [1, 2, 3, 4, 5]
smallArray.insert(0, at: 0) // Fast enough with only 5 elements
var largeArray = Array(1...10000)
// This could cause a momentary freeze in your UI:
largeArray.insert(0, at: 0)
If you find yourself frequently adding items to the beginning of an array, you might want to consider using a different collection type like Deque
(available in Swift 5.8+) or redesigning your algorithm.
Similarly, searching through unsorted arrays can be inefficient. When you use methods like firstIndex(of:)
or contains(_:)
, Swift has to check each element one by one until it finds a match. For larger arrays, consider whether sorting the array first (if you'll search it multiple times) or using a different collection type like Set
(if you only care about uniqueness and order doesn't matter) might be more appropriate for your needs.
ii. Value vs reference behavior - Why arrays copy their data only when needed and how this impacts your code
Swift arrays use a clever optimization called "copy-on-write" that significantly improves performance while maintaining the safety of value semantics. When you assign an array to a new variable or pass it to a function, Swift doesn't immediately create a full copy of the array's contents. Instead, it creates a new array variable that references the same underlying storage as the original. Only when you modify one of the arrays does Swift create a separate copy of the contents.
This behavior combines the best of both worlds: arrays behave like values (changes to one copy don't affect others) while avoiding unnecessary copying operations. Consider this example:
var originalArray = [1, 2, 3, 4, 5]
var newArray = originalArray // No copying happens here
// At this point, both variables share the same storage
// When we modify newArray, Swift creates a copy before changing it
newArray.append(6)
print(originalArray) // [1, 2, 3, 4, 5] - Unchanged
print(newArray) // [1, 2, 3, 4, 5, 6] - Modified
In SwiftUI, this behavior is particularly important. When you pass an array to a child view, you're not creating an expensive copy. However, if your arrays contain reference types (classes rather than structs), remember that the array elements themselves still follow reference semantics:
class Person { var name: String; init(name: String) { self.name = name } }
var people1 = [Person(name: "Taylor"), Person(name: "John")]
var people2 = people1 // Array container uses copy-on-write
// But the Person objects inside are references
people2[0].name = "Changed"
// Both arrays reflect the change because they contain references
// to the same Person objects
print(people1[0].name) // "Changed"
iii. Memory usage tips - Simple ways to make your arrays use less memory in your SwiftUI apps
Managing memory efficiently is crucial, especially in iOS apps where resources can be constrained. Here are practical techniques to optimize memory usage with arrays:
When you need to clear an array temporarily but plan to refill it soon, use removeAll(keepingCapacity: true)
to maintain the allocated memory:
var cachedResults = [ComplexCalculationResult]()
// Fill the array with 1000 items...
// Later, when refreshing:
cachedResults.removeAll(keepingCapacity: true) // Keeps allocated space
// Refill the array...
For arrays that grow incrementally, pre-allocate space when you know the eventual size:
var userNames = [String]()
userNames.reserveCapacity(1000) // Allocates space for 1000 items
// Now adding items won't require frequent reallocations
Be mindful of array slices. While ArraySlice
is efficient because it doesn't copy data, it maintains a reference to the original array, potentially keeping a large array in memory:
let largeArray = Array(1...10000)
let firstTen = largeArray.prefix(10) // Creates an ArraySlice
// largeArray is still in memory as long as firstTen exists
// If you only need the small part, convert to a new array
let firstTenCopy = Array(firstTen) // Now largeArray can be deallocated
In SwiftUI, when displaying large collections, use lazy loading views to reduce memory usage:
ScrollView {
LazyVStack { // Only loads visible elements
ForEach(hugeArray, id: \.self) { item in
ItemRow(item: item)
}
}
}
B. Common Problems and Solutions
i. Handling out-of-bounds errors - Safe ways to access array elements without crashing your app
Array index out of bounds errors are among the most common crashes in Swift apps. These occur when you attempt to access an element at an index that doesn't exist. Instead of direct subscripting, consider these safer approaches:
Add a safe subscript extension to Array:
extension Collection {
subscript(safe index: Index) -> Element? {
return indices.contains(index) ? self[index] : nil
}
}
// Usage:
let array = ["a", "b", "c"]
if let element = array[safe: 10] {
print(element)
} else {
print("Index is out of bounds")
}
Use optional chaining with the indices
property:
let array = ["a", "b", "c"]
let index = 5
if array.indices.contains(index) {
let element = array[index]
print(element)
} else {
print("Index is out of bounds")
}
In SwiftUI, this is especially important when dealing with user-driven navigation or selection:
struct DetailView: View {
let items: [Item]
let selectedIndex: Int
var body: some View {
if items.indices.contains(selectedIndex) {
ItemDetailView(item: items[selectedIndex])
} else {
Text("Item not found")
}
}
}
ii. SwiftUI array update issues - Potential issues and solutions when working with arrays in your UI
SwiftUI relies on identity to track changes in collections. When updating arrays, ensure your elements are properly identifiable to avoid UI glitches and performance problems:
Always use Identifiable
or explicit id
parameters with ForEach
:
// Preferred: Using Identifiable
struct Task: Identifiable {
let id = UUID()
var title: String
}
ForEach(tasks) { task in
TaskRow(task: task)
}
// Alternative: Using id parameter
ForEach(items, id: \.uniqueId) { item in
ItemRow(item: item)
}
When modifying arrays in SwiftUI, create new arrays rather than mutating in place:
// Instead of this (can cause SwiftUI update problems):
@State private var tasks = [Task]()
func completeTask(at index: Int) {
tasks[index].isCompleted = true // Modifying in place
}
// Do this (creates a new array, properly triggering updates):
@State private var tasks = [Task]()
func completeTask(at index: Int) {
var updatedTasks = tasks
updatedTasks[index].isCompleted = true
tasks = updatedTasks // Assigns a new array
}
For complex operations like filtering, sorting, or mapping, use functional approaches that return new arrays:
// This creates a new array, properly triggering SwiftUI updates
tasks = tasks.filter { !$0.isCompleted }
iii. Working with arrays of custom objects - Ensuring your arrays work correctly when they contain your own data types
When using arrays of custom objects in SwiftUI, proper type design ensures smooth integration:
Implement Identifiable
for SwiftUI collections:
struct Contact: Identifiable {
let id = UUID()
var name: String
var email: String
}
// Now ForEach works naturally
ForEach(contacts) { contact in
ContactRow(contact: contact)
}
For value comparison and SwiftUI diffing, implement Equatable
:
struct Contact: Identifiable, Equatable {
let id = UUID()
var name: String
var email: String
static func == (lhs: Contact, rhs: Contact) -> Bool {
return lhs.id == rhs.id &&
lhs.name == rhs.name &&
lhs.email == rhs.email
}
}
If your custom types contain reference types (classes), be careful about reference sharing:
class ProfileImage {
var data: Data
init(data: Data) { self.data = data }
}
struct User {
let id = UUID()
var name: String
var profileImage: ProfileImage // Reference type
}
// Be careful - multiple User structs might share the same ProfileImage
// Changes to one will affect all
Consider making deep copies when needed:
extension User {
func copyWithNewImage() -> User {
let imageCopy = ProfileImage(data: self.profileImage.data)
var userCopy = self
userCopy.profileImage = imageCopy
return userCopy
}
}
By keeping these practices in mind, you'll avoid common pitfalls and create more efficient, reliable SwiftUI applications that handle arrays effectively.
6. Practical Examples
Moving from theoretical concepts to hands-on applications is where you truly solidify your understanding of Swift arrays. This section brings together everything we've covered—from basic array operations to performance considerations—into complete, real-world examples that you can use as templates for your own projects. By examining these practical implementations, you'll see how arrays form the foundation of common iOS app features and gain insight into the design patterns that professional developers use when building production applications. These examples serve as both a synthesis of previous lessons and a launchpad for your own creative development with SwiftUI.
This section features two complete SwiftUI projects that demonstrate arrays in real-world applications, both created entirely with Claude 3.7 Sonnet based on the concepts we've covered. The ToDoList project showcases a foundational implementation of arrays in a task management application. It demonstrates essential techniques for model creation, task manipulation, and basic state management—perfect for understanding how our array concepts come together in a practical context. The AdvancedToDoList project builds on these basics with sophisticated features like data persistence, filtering capabilities, and more complex state management patterns. This project illustrates how array operations scale to meet more demanding requirements in production-quality applications. You can explore the source code to see practical implementations of the techniques discussed throughout this tutorial, or challenge yourself to optimize these projects further by applying performance best practices. Each project is fully documented to highlight key array implementation details and design decisions.
A. Building a To-Do List App
i. Model creation with arrays - The foundation of any data-driven application is a well-designed model layer, and to-do list apps are no exception. Creating a robust task model involves careful consideration of data structure, identity management, and state handling to ensure your application behaves predictably. Swift's strong typing combined with protocols like Identifiable
and Codable
enables you to create models that are both type-safe and easily serializable. This section walks through designing a complete task management model that serves as the backbone for our to-do application, focusing on array-based collections that support filtering, sorting, and persistence while maintaining clean integration with SwiftUI's state management system. This thoughtful model design establishes a solid foundation for building a responsive, maintainable to-do app.
ii. Adding and removing tasks - The heart of any to-do app lies in its ability to smoothly handle task creation and deletion. SwiftUI's reactive nature makes these operations straightforward conceptually, but implementing them with proper animation, error handling, and user feedback requires careful attention to detail. This section explores the complete workflow for managing tasks, from designing intuitive input forms and validation logic to implementing swipe-to-delete functionality and batch operations. You'll learn how to build interfaces that make task management feel natural and responsive, with carefully choreographed transitions that enhance the user experience. These patterns form the foundation for virtually any collection-based app, making them valuable beyond just to-do list applications.
iii. Persisting array data - A truly useful to-do app must persist tasks between app launches, ensuring users don't lose their data when they close the application. Swift offers several approaches to data persistence, each with different tradeoffs in terms of complexity, flexibility, and performance. This section explores implementing persistence for array-based data models using three common approaches: UserDefaults for simple storage, document-based storage with FileManager, and SwiftData for more complex database-like capabilities. You'll learn best practices for serializing and deserializing array data, implementing error handling for robust storage operations, and designing a clean architecture that separates persistence concerns from your view and model logic. These persistence patterns form an essential component of production-quality iOS applications.
Learn with videos and source files. Available to Pro subscribers only.
Purchase includes access to 50+ courses, 320+ premium tutorials, 300+ hours of videos, source files and certificates.
Templates and source code
Download source files
Download the videos and assets to refer and learn offline without interuption.
Design template
Source code for all sections
Video files, ePub and subtitles
Browse all downloads
1
Building Your iOS Development Foundation
Master the fundamentals of Swift programming with hands-on examples designed for beginners and experienced developers alike
2
Print Statements Debugging
Unlock the invisible processes in SwiftUI with strategic print statements that illuminate state changes, view lifecycles, and data flow
18:04
3
Comments: Documentation Waypoints in Your SwiftUI Codebase
Transform your code from mysterious instructions to a comprehensive narrative with strategic comments that explain the why
14:39
4
Variables and Constants
Learn when and how to use variables and constants to write safer, more efficient SwiftUI code
11:37
5
Strings and Interpolation
Learn essential string operations in Swift: Build better iOS apps with efficient text handling techniques
13:22
6
Swift Operators: The Foundation of SwiftUI Logic
Building powerful iOS apps through the language of operations
7
Unary Operators
Mastering the elegant simplicity of unary operators for cleaner, more expressive SwiftUI code that transforms your UI with minimal syntax
8
Binary Operators
Master the two-operand symbols that transform complex interface logic into concise, readable declarations
9
Arithmetic Operators
Learn how to implement and optimize arithmetic operations in SwiftUI, from basic calculations to complex mathematical interfaces
10
If-Else and Comparison Operators
Building Dynamic SwiftUI: Mastering If-Else and Comparison Operators
11
Logical Operators
Master SwiftUI's logical operators: Building intelligent iOS apps with robust decision-making systems
12
Ternary Operators
Use the power of Swift's ternary conditional operator to create dynamic, responsive interfaces with minimal code in SwiftUI
13
Blocks and Scope
A comprehensive guide to writing clean, organized code through proper variable management and state control
10:22
14
Swift Collections: Arrays, Sets, and Dictionaries Overview
Organize Your Data Effectively: Learn How to Choose and Optimize the Perfect Collection Type for Your SwiftUI Applications
15
Swift Arrays: The Basics Part 1
Master essential array operations and manipulations to build a solid foundation for iOS development
16
Swift Arrays: Best Practices in SwiftUI Part 2
Integrate arrays with SwiftUI, optimize performance, and implement professional-grade patterns for production apps
Meet the instructor
We all try to be consistent with our way of teaching step-by-step, providing source files and prioritizing design in our courses.
Sourasith Phomhome
UI Designer
Designer at Design+Code
19 courses - 74 hours
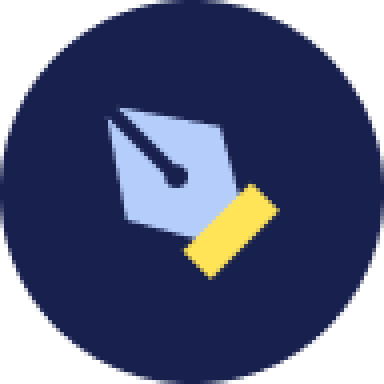
Design Multiple Apps with Figma and AI
In this course, you’ll learn to design multiple apps using Figma and AI-powered tools, tackling a variety of real-world UI challenges. Each week, a new episode will guide you through a different design, helping you master essential UI/UX principles and workflows
4 hrs
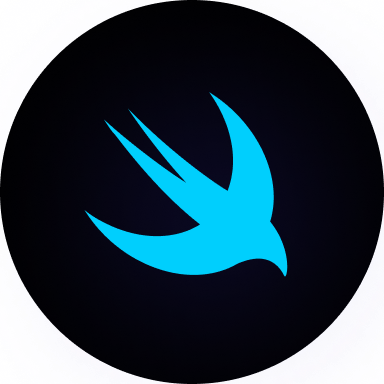
SwiftUI Fundamentals Handbook
A comprehensive guide to mastering Swift programming fundamentals, designed for aspiring iOS developers. This handbook provides a structured approach to learning Swift's core concepts, from basic syntax to advanced programming patterns. Through carefully sequenced chapters, readers will progress from essential programming concepts to object-oriented principles, building a solid foundation for SwiftUI development. Each topic includes practical examples and clear explanations, ensuring a thorough understanding of Swift's capabilities. This handbook serves as both a learning resource and a reference guide, covering fundamental concepts required for modern iOS development. Topics are presented in a logical progression, allowing readers to build their knowledge systematically while gaining practical programming skills.
1 hrs
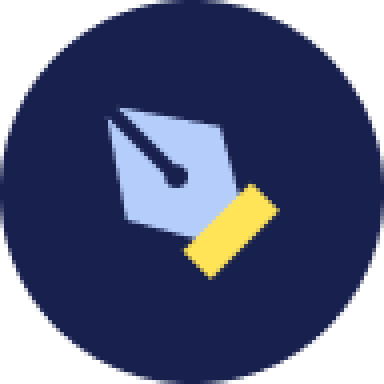
Design and Code User Interfaces with Galileo and Claude AI
In this course, you’ll learn how to use AI tools to make UI/UX design faster and more efficient. We’ll start with Galileo AI to create basic designs, providing a solid foundation for your ideas. Next, we’ll refine these designs in Figma to match your personal style, and finally, we’ll use Claude AI to turn them into working code—eliminating the need for traditional prototyping.
4 hrs
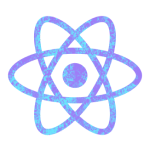
Build a React Native app with Claude AI
This comprehensive course explores the integration of cutting-edge AI tools into the React Native development workflow, revolutionizing the approach to mobile application creation. Participants will learn to leverage AI-powered platforms such as Claude and Locofy to expedite coding processes, enhance problem-solving capabilities, and optimize productivity.
13 hrs
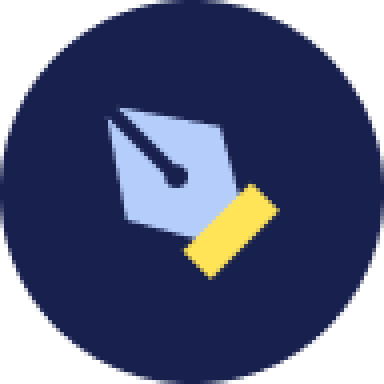
Design and Prototype for iOS 18
Design and Prototype for iOS 18 is an immersive course that equips you with the skills to create stunning, user-friendly mobile applications. From mastering Figma to understanding iOS 18's latest design principles, you'll learn to craft two real-world apps - a Car Control interface and an AI assistant.
3 hrs
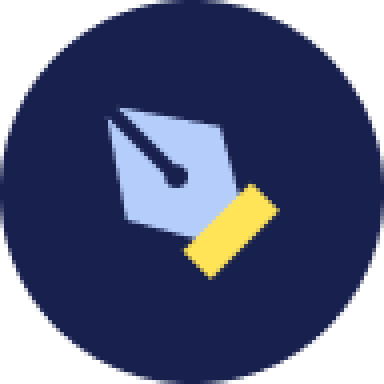
Master Responsive Layouts in Figma
Creating responsive layouts is a must-have skill for any UI/UX designer. With so many different devices and screen sizes, designing interfaces that look great and work well on all platforms is necessary. Mastering this skill will make you stand out in the field. In this course, we'll start from scratch to create this beautiful design using Figma. You'll learn how to make layouts that are easy to use and work well on any device. We'll cover key concepts and tools to help you master responsive design in Figma.
2 hrs
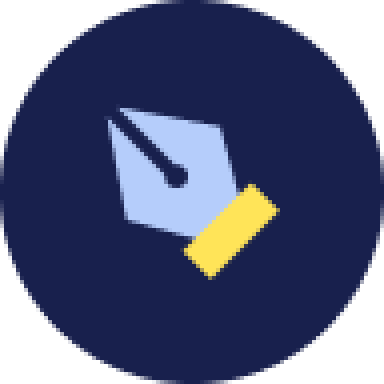
UI UX Design with Mobbin and Figma
Mobbin is a powerful tool for UI/UX designers seeking inspiration and innovative design solutions. This platform offers a vast collection of real-world mobile app designs, providing a treasure trove of UI elements and layouts.
2 hrs
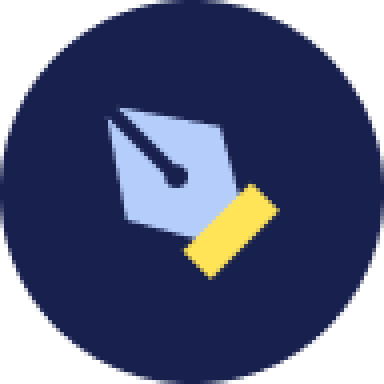
3D UI Interactive Web Design with Spline
Learn to create 3D designs and UI interactions such as 3D icons, UI animations, components, variables, screen resize, scrolling interactions, as well as exporting, optimizing, and publishing your 3D assets on websites
3 hrs
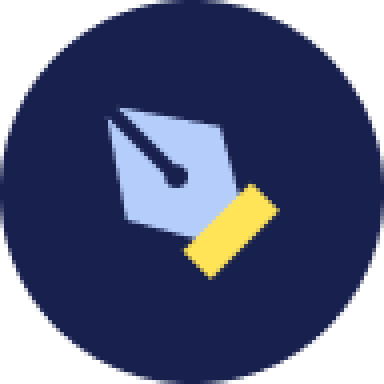
Design and Prototype for iOS 17 in Figma
Crafting engaging experiences for iOS 17 and visionOS using the Figma design tool. Learn about Figma's new prototyping features, Dev Mode, variables and auto layout.
6 hrs
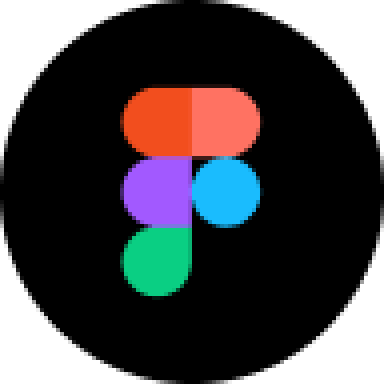
Design and Prototype Apps with Midjourney
A comprehensive course on transforming Midjourney concepts into interactive prototypes using essential design techniques and AI tools
8 hrs
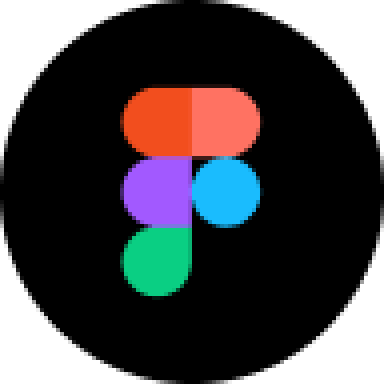
iOS Design with Midjourney and Figma
Learn the fundamentals of App UI design and master the art of creating beautiful and intuitive user interfaces for mobile applications
1 hrs
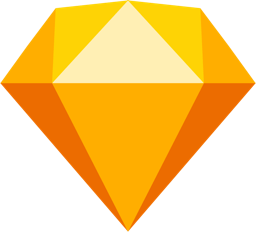
UI Design for iOS, Android and Web in Sketch
Create a UI design from scratch using Smart Layout, Components, Prototyping in Sketch app
1 hrs
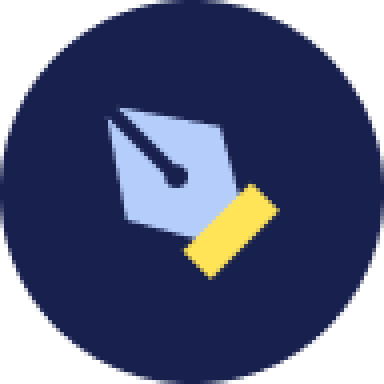
UI Design a Camera App in Figma
Design a dark, vibrant and curvy app design from scratch in Figma. Design glass icons, lens strokes and realistic buttons.
1 hrs
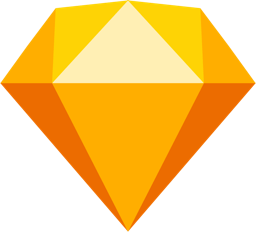
UI Design for iOS 16 in Sketch
A complete guide to designing for iOS 16 with videos, examples and design files
3 hrs
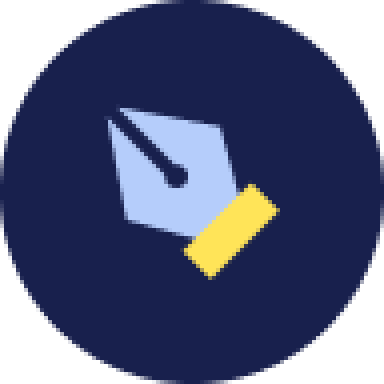
Prototyping in Figma
Learn the basics of prototyping in Figma by creating interactive flows from custom designs
1 hrs
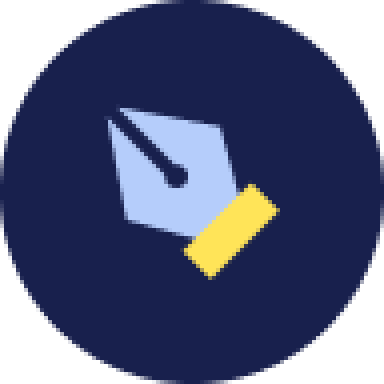
UI Design Quick Websites in Figma
Learn how to design a portfolio web UI from scratch in Figma
1 hrs
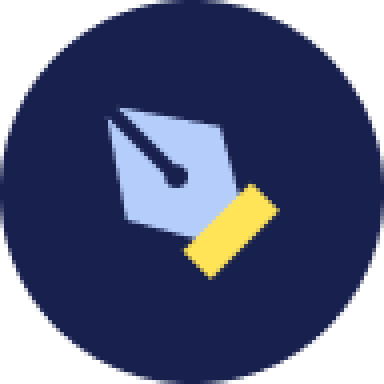
UI Design Android Apps in Figma
Design Android application UIs from scratch using various tricks and techniques in Figma
2 hrs
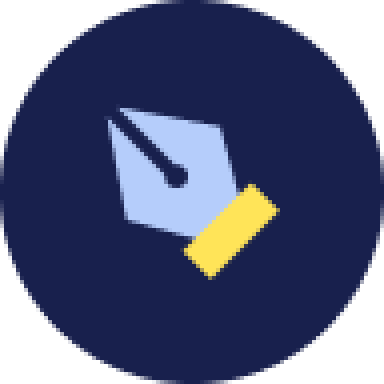
UI Design Quick Apps in Figma
Design application UIs from scratch using various tricks and techniques in Figma
12 hrs
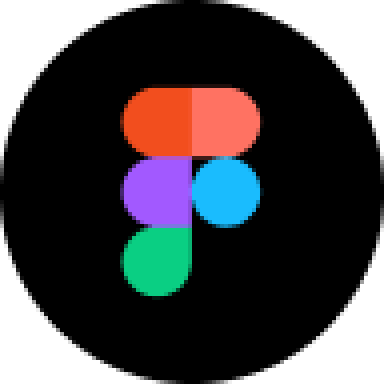
Figma Handbook
A comprehensive guide to the best tips and tricks in Figma
6 hrs