Intro to Firebase
Add to favorites
Learn about Firebase and set it up in your React project
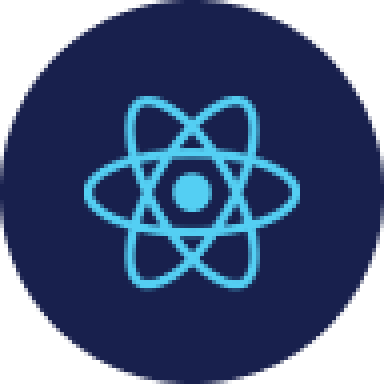
Advanced React Hooks Handbook
1
Intro to Firebase
6:59
2
Firebase Auth
11:59
3
Firestore
10:51
4
Firebase Storage
6:40
5
Serverless Email Sending
10:02
6
Geocoding with Mapbox
9:24
7
Contentful Part 1
4:59
8
Contentful Part 2
8:52
9
Gatsby and Shopify Part 1
5:20
10
Gatsby and Shopify Part 2
13:21
11
Gatsby and Shopify Part 3
14:32
12
Creating Stripe Account and Product
5:18
13
Adding Stripe.js to React
5:54
14
Stripe Checkout Client Only
18:18
15
PayPal Checkout
31:21
CodeSandbox link
You can find the full code for this tutorial at https://codesandbox.io/s/intro-to-firebase-gtnjo.
Create a Firebase project
Head over to the Firebase Console and click on Create a project.
Follow the three easy steps to create your first Firebase project:
- Give a name to your project.
- Enable Google Analytics if you wish to (you can always enable it later). For now, I'll disable it.
- Wait for your project to be created and click on Continue.
Add you app
After your project has been created, you'll land on your project's Firebase console. There'll be a banner like this one, inviting you to add Firebase to your app. Click on the Web option - the button with the brackets.
Give your app a nickname. If you wish to host your app with Firebase Hosting, check the box. However, I won't host it on Firebase, so I won't check it. Then, click on Register app.
At step 2, there'll be a script with all your config variables. We will get back to it later. Click on Continue to console.
Add the Firebase SDK to your project
Next, we'll head over to React project. For the purpose of this tutorial, I'll be using Code Sandbox. Creating a new project and adding a dependency is pretty simple. Under the Dependencies tab on the right, search for Firebase and click the first result.
If your React app is on your local computer, you can install the Firebase SDK through the terminal with the following command:
npm i --save firebase
Set up the Firebase configuration
Next, we'll need to set up the configuration in our React project. In other words, we need to add the private keys from Firebase into our React project. The private keys are like an address to your Firebase project and will let React and Firebase communicate with each other to save the data at the right place.
Let's create a new file called firebase.js, where all of our Firebase configuration will be.
First, let's import Firebase at the top.
// firebase.js
import firebase from 'firebase'
Next, we'll need to copy the configuration keys from our Firebase project. At the right, click on the gear icon next the Project Overview, then on Project settings.
Scroll down the Your apps section. There, you'll see your Firebase SDK snippet. Copy everything from Your web app's Firebase configuration to firebase.initializeApp(firebaseConfig).
Head back to your React project and paste everything you just copied into the firebase.js file. We'll need to change a few things here.
Firstly, if you're not using Code Sandbox and you commit your project to Github or any similar platform, remember to never hard-code your private keys into your project as it is a big security flaw. We'll need to create a .env file and add all our keys there.
// .env
REACT_APP_FIREBASE_API_KEY=YOUR_API_KEY_HERE
REACT_APP_FIREBASE_AUTHDOMAIN=YOUR_AUTH_DOMAIN_HERE
REACT_APP_FIREBASE_PROJECT_ID=YOUR_PROJECT_HERE
REACT_APP_FIREBASE_STORAGE_BUCKET=YOUR_STORAGE_BUCKET_HERE
REACT_APP_FIREBASE_MESSAGING_SENDER_ID=YOUR_MESSAGING_SENDER_ID_HERE
REACT_APP_FIREBASE_APP_ID=YOUR_FIREBASE_APP_ID
Then, create a .gitignore file and add .env to it. This will tell the terminal to ignore the .env file when you're committing to Github.
// .gitignore
.env
Back to our firebase.js file, we'll change all the hard-coded private keys to the ones we added in the .env file.
var firebaseConfig = {
apiKey: process.env.REACT_APP_FIREBASE_API_KEY,
authDomain: process.env.REACT_APP_FIREBASE_AUTHDOMAIN,
projectId: process.env.REACT_APP_FIREBASE_PROJECT_ID,
storageBucket: process.env.REACT_APP_FIREBASE_STORAGE_BUCKET,
messagingSenderId: process.env.REACT_APP_FIREBASE_MESSAGING_SENDER_ID,
appId: process.env.REACT_APP_FIREBASE_APP_ID
};
Note: In Code Sandbox, you can store your API keys under the Server Tab on the right side. However, to see the Server Menu, you must fork an imported Sandbox.
Next, before initializing the app, we need to make sure that the window isn't undefined first. So replace the firebase.initializeApp(firebaseConfig) line with this code:
let instance
export default function getFirebase() {
if (typeof window !== "undefined") {
if (instance) return instance
instance = firebase.initializeApp(firebaseConfig);
return instance
}
return null
}
In the code snippet above, we are creating a function that will make sure that the window isn't undefined - if it is, it'll return null instead of initializing Firebase for nothing. If there's a window, it'll return the instance of the Firebase app that we initialized.
Use Firebase
To use Firebase in your project, import the getFirebase() function at the top the file.
import getFirebase from "./firebase"
Make sure to check that we get an instance of Firebase before doing any action with Firebase.
let firebase = getFirebase()
if (firebase) {
// Do something
}
Congratulations! You just set up Firebase in your React application! You can now head over to the Firebase Auth, Firestore and Firebase Storage sections of this handbook to learn how to use a specific Firebase service in your application.
Learn with videos and source files. Available to Pro subscribers only.
Purchase includes access to 50+ courses, 320+ premium tutorials, 300+ hours of videos, source files and certificates.
Templates and source code
Download source files
Download the videos and assets to refer and learn offline without interuption.
Design template
Source code for all sections
Video files, ePub and subtitles
Browse all downloads
1
Intro to Firebase
Learn about Firebase and set it up in your React project
6:59
2
Firebase Auth
Set up authentication in your React application using Firebase Auth
11:59
3
Firestore
Enable Firestore as your database in your React application
10:51
4
Firebase Storage
Enable Firebase Storage in your application to store photos or videos from users
6:40
5
Serverless Email Sending
Use EmailJS to send an email without backend
10:02
6
Geocoding with Mapbox
Create an address autocomplete with Mapbox's Geocoding API
9:24
7
Contentful Part 1
Create a Contentful account, add a model and content
4:59
8
Contentful Part 2
How to use the Content Delivery API to fetch data from Contentful
8:52
9
Gatsby and Shopify Part 1
Create a Shopify store, generate a password and add products to the store
5:20
10
Gatsby and Shopify Part 2
Connect Shopify to Gatsby and display all products
13:21
11
Gatsby and Shopify Part 3
Create a seamless checkout experience with Shopify and Gatsby
14:32
12
Creating Stripe Account and Product
Start integrating with Stripe and set up an account, product and price
5:18
13
Adding Stripe.js to React
Loading Stripe.js library to your React client application
5:54
14
Stripe Checkout Client Only
Accept payment with Stripe Checkout without backend
18:18
15
PayPal Checkout
Integrate online payment with PayPal
31:21
Meet the instructor
We all try to be consistent with our way of teaching step-by-step, providing source files and prioritizing design in our courses.
Stephanie Diep
iOS and Web developer
Developing web and mobile applications while learning new techniques everyday
7 courses - 36 hours
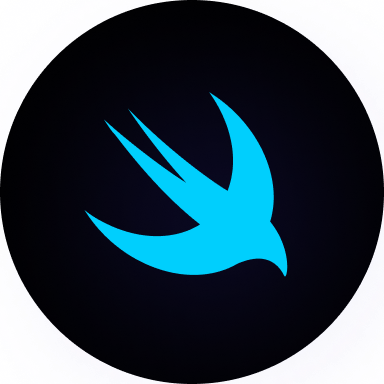
Build Quick Apps with SwiftUI
Apply your Swift and SwiftUI knowledge by building real, quick and various applications from scratch
11 hrs
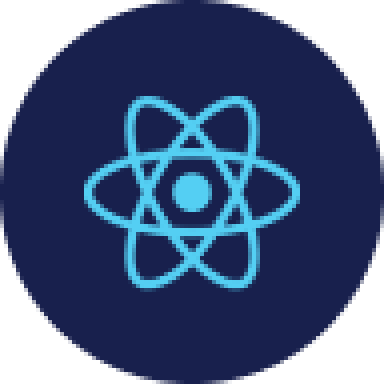
Advanced React Hooks Handbook
An extensive series of tutorials covering advanced topics related to React hooks, with a main focus on backend and logic to take your React skills to the next level
3 hrs
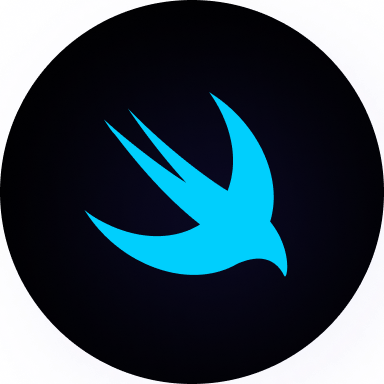
SwiftUI Concurrency
Concurrency, swipe actions, search feature, AttributedStrings and accessibility were concepts discussed at WWDC21. This course explores all these topics, in addition to data hosting in Contentful and data fetching using Apollo GraphQL
3 hrs
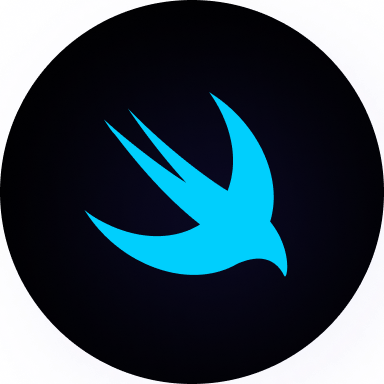
SwiftUI Combine and Data
Learn about Combine, the MVVM architecture, data, notifications and performance hands-on by creating a beautiful SwiftUI application
3 hrs
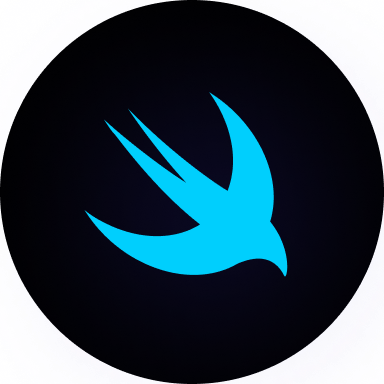
SwiftUI Advanced Handbook
An extensive series of tutorials covering advanced topics related to SwiftUI, with a main focus on backend and logic to take your SwiftUI skills to the next level
4 hrs
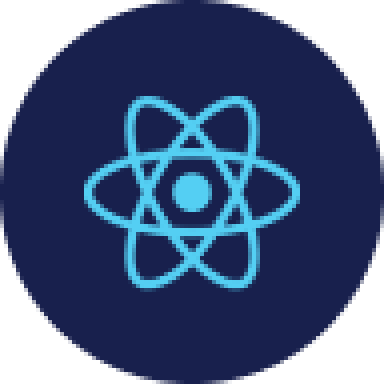
React Hooks Handbook
An exhaustive catalog of React tutorials covering hooks, styling and some more advanced topics
5 hrs
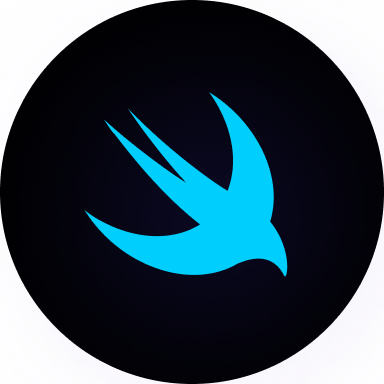
SwiftUI Handbook
A comprehensive series of tutorials covering Xcode, SwiftUI and all the layout and development techniques
7 hrs