Gatsby and Shopify Part 3
Add to favorites
Create a seamless checkout experience with Shopify and Gatsby
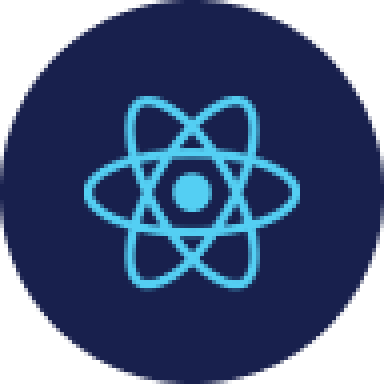
Advanced React Hooks Handbook
1
Intro to Firebase
6:59
2
Firebase Auth
11:59
3
Firestore
10:51
4
Firebase Storage
6:40
5
Serverless Email Sending
10:02
6
Geocoding with Mapbox
9:24
7
Contentful Part 1
4:59
8
Contentful Part 2
8:52
9
Gatsby and Shopify Part 1
5:20
10
Gatsby and Shopify Part 2
13:21
11
Gatsby and Shopify Part 3
14:32
12
Creating Stripe Account and Product
5:18
13
Adding Stripe.js to React
5:54
14
Stripe Checkout Client Only
18:18
15
PayPal Checkout
31:21
We created our Shopify store and connected the products data to our Gatsby website . In this last section of the Gatsby and Shopify series, we'll cover the checkout process. We'll be using the shopify-buy npm package from Shopify to create a seamless checkout experience.
Purchase includes access to 50+ courses, 320+ premium tutorials, 300+ hours of videos, source files and certificates.
Learn with videos and source files. Available to Pro subscribers only.
Purchase includes access to 50+ courses, 320+ premium tutorials, 300+ hours of videos, source files and certificates.
Templates and source code
Download source files
Download the videos and assets to refer and learn offline without interuption.
Design template
Source code for all sections
Video files, ePub and subtitles
Browse all downloads
1
Intro to Firebase
Learn about Firebase and set it up in your React project
6:59
2
Firebase Auth
Set up authentication in your React application using Firebase Auth
11:59
3
Firestore
Enable Firestore as your database in your React application
10:51
4
Firebase Storage
Enable Firebase Storage in your application to store photos or videos from users
6:40
5
Serverless Email Sending
Use EmailJS to send an email without backend
10:02
6
Geocoding with Mapbox
Create an address autocomplete with Mapbox's Geocoding API
9:24
7
Contentful Part 1
Create a Contentful account, add a model and content
4:59
8
Contentful Part 2
How to use the Content Delivery API to fetch data from Contentful
8:52
9
Gatsby and Shopify Part 1
Create a Shopify store, generate a password and add products to the store
5:20
10
Gatsby and Shopify Part 2
Connect Shopify to Gatsby and display all products
13:21
11
Gatsby and Shopify Part 3
Create a seamless checkout experience with Shopify and Gatsby
14:32
12
Creating Stripe Account and Product
Start integrating with Stripe and set up an account, product and price
5:18
13
Adding Stripe.js to React
Loading Stripe.js library to your React client application
5:54
14
Stripe Checkout Client Only
Accept payment with Stripe Checkout without backend
18:18
15
PayPal Checkout
Integrate online payment with PayPal
31:21
Meet the instructor
We all try to be consistent with our way of teaching step-by-step, providing source files and prioritizing design in our courses.
Stephanie Diep
iOS and Web developer
Developing web and mobile applications while learning new techniques everyday
7 courses - 36 hours
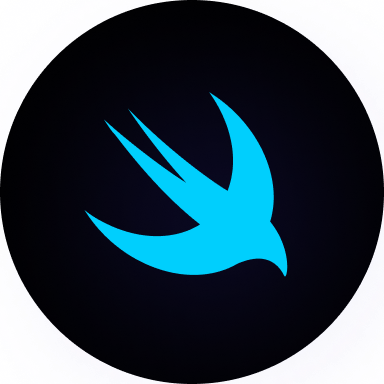
Build Quick Apps with SwiftUI
Apply your Swift and SwiftUI knowledge by building real, quick and various applications from scratch
11 hrs
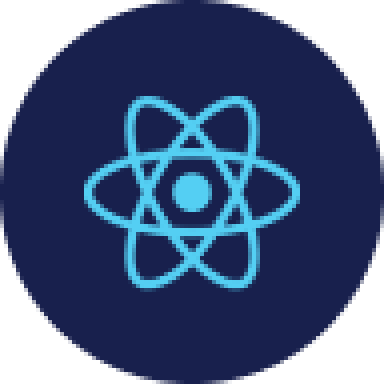
Advanced React Hooks Handbook
An extensive series of tutorials covering advanced topics related to React hooks, with a main focus on backend and logic to take your React skills to the next level
3 hrs
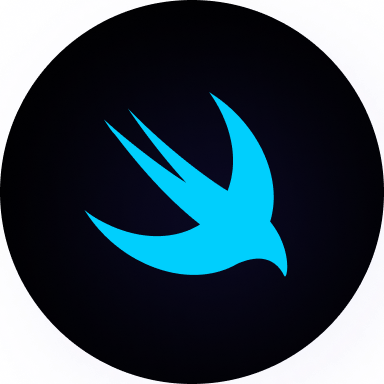
SwiftUI Concurrency
Concurrency, swipe actions, search feature, AttributedStrings and accessibility were concepts discussed at WWDC21. This course explores all these topics, in addition to data hosting in Contentful and data fetching using Apollo GraphQL
3 hrs
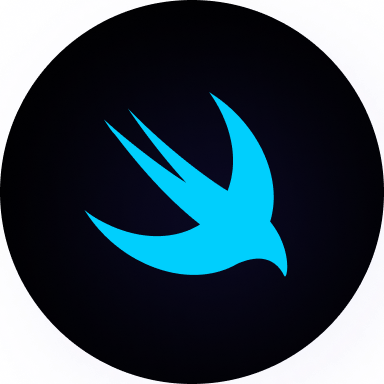
SwiftUI Combine and Data
Learn about Combine, the MVVM architecture, data, notifications and performance hands-on by creating a beautiful SwiftUI application
3 hrs
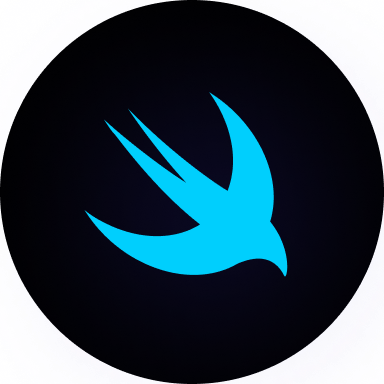
SwiftUI Advanced Handbook
An extensive series of tutorials covering advanced topics related to SwiftUI, with a main focus on backend and logic to take your SwiftUI skills to the next level
4 hrs
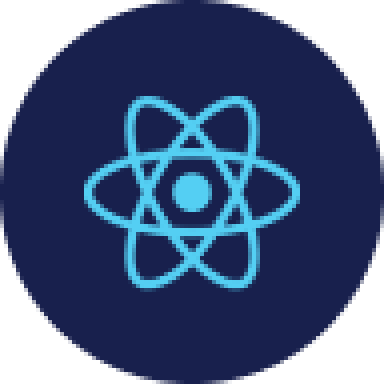
React Hooks Handbook
An exhaustive catalog of React tutorials covering hooks, styling and some more advanced topics
5 hrs
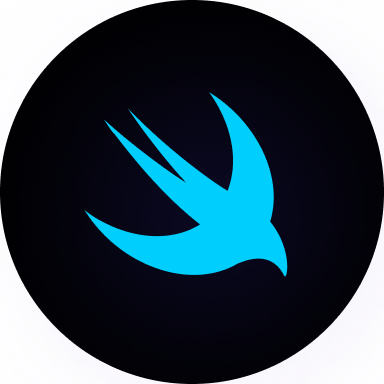
SwiftUI Handbook
A comprehensive series of tutorials covering Xcode, SwiftUI and all the layout and development techniques
7 hrs