Sketch Plugin Project
Add to favorites
Sketch Plugin Environment and the Package Manager
Sketch is the design tool that was launched in the late 2000’s and spread like wildfire in the design community due to its simplicity, focus, and ease of use. It is mainly but not solely focused on user interface design. If you are here, you probably know it by heart and want to make the Sketch workflow even more productive using the plugin API.
Your first stop in your journey should be the Sketch Developer Portal where you will find the documentation, the forum, and examples. In this chapter, we are going to focus on building key parts of Angle from scratch. In this section, we are going to get your project setup and register the first menubar command.
Purchase includes access to 50+ courses, 320+ premium tutorials, 300+ hours of videos, source files and certificates.
Templates and source code
Download source files
Download the videos and assets to refer and learn offline without interuption.
Design template
Source code for all sections
Video files, ePub and subtitles
Browse all downloads
1
Sketch Plugin Project
Sketch Plugin Environment and the Package Manager
16:42
2
Angle Class
Using a Prototype to Reuse Code
20:04
3
Prototyping Perspective
Using Core Image to Prototype the Transform
7:25
4
Apply Mockup
Apply Perspective to an Artboard
17:15
5
Rotation and Mirror
Generate Stateful Commands
24:51
6
Working with Symbols
Investigating Sketch Classes
13:35
7
Dialog and Selections
Building a Cocoa UI to Handle Multi-Selection
31:10
Meet the instructor
We all try to be consistent with our way of teaching step-by-step, providing source files and prioritizing design in our courses.
Tiago Mergulhão
Interaction Designer
Pixel-crafting experiences for touch and click on a daily basis
3 courses - 15 hours
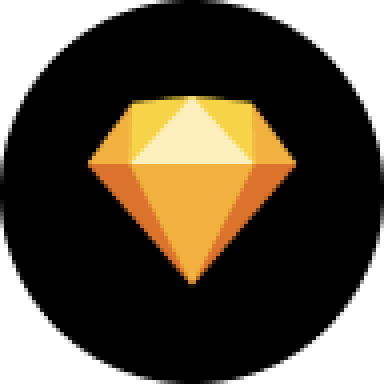
Create a Sketch Plugin
Overview of the Sketch Developer Portal by making you workflow even more productive using the plugin API
2 hrs

Swift Advanced
Learn Swift a robust and intuitive programming language created by Apple for building apps for iOS, Mac, Apple TV and Apple Watch
9 hrs
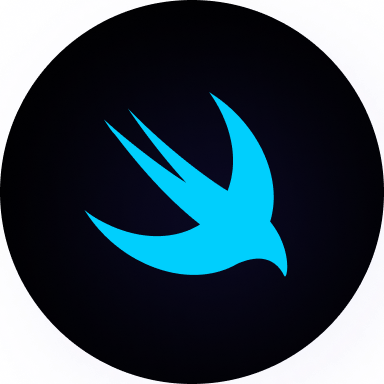
Learn Swift
Learn Swift a robust and intuitive programming language created by Apple for building apps for iOS, Mac, Apple TV and Apple Watch
4 hrs